What is Pyodbc? Installation to Implementation
Whether a beginner or an experienced developer, you’ll learn to integrate pyODBC into your projects, enabling efficient data manipulation and retrieval. pyODBC has a large user base, with 1,30,000 downloads/month. Based on a survey published, pyODBC is preferred by 23% of all the respondents. Regarding the popularity of pyODBC, its Github repository is stared 10,000 times with the same number of forks. This data suggests that pyODBC is still widely used, irrespective of its other alternatives.
Table of Contents:
- What is pyODBC?
- How to Install pyODBC?
- pyODBC Syntax and Examples: 10 Essential Code Snippets
- How to Link pyODBC with Various Databases?
- Troubleshooting pyODBC Errors
- Best Practices in pyODBC to Run Queries Faster
- Advantages of Using pyODBC
- What are the Alternatives to pyODBC?
- Conclusion
Kickstart your Python journey with our Python Tutorial for absolute beginners
{
“@context”: “https://schema.org”,
“@type”: “VideoObject”,
“name”: “Python Course | Python Tutorial For Beginners | Python Training | Intellipaat”,
“description”: “What is Pyodbc? Installation to Implementation”,
“thumbnailUrl”: “https://img.youtube.com/vi/uJGIp1SwG1g/hqdefault.jpg”,
“uploadDate”: “2023-08-29T08:00:00+08:00”,
“publisher”: {
“@type”: “Organization”,
“name”: “Intellipaat Software Solutions Pvt Ltd”,
“logo”: {
“@type”: “ImageObject”,
“url”: “https://intellipaat.com/blog/wp-content/themes/intellipaat-blog-new/images/logo.png”,
“width”: 124,
“height”: 43
}
},
“embedUrl”: “https://www.youtube.com/embed/uJGIp1SwG1g”
}
What is pyODBC?
pyODBC is a vital Python module that facilitates smooth interaction with various databases through the Open Database Connectivity (ODBC) interface. It allows Python programs to communicate effectively with various database management systems, including SQL Server, Oracle, MySQL, and many more.
- pyODBC’s significance lies in its capability to provide a generalized method for accessing and manipulating data on disparate database platforms.
- Its adoption facilitates the development process by eliminating the need to learn specific database drivers for each system, promoting code reusability, and reducing complexity.
- The key advantage of pyODBC is its ability to bridge the gap between Python’s user-friendly syntax and the complexities of database systems.
- Developers can utilize the power of Python’s data manipulation capabilities while efficiently interfacing with databases to retrieve, alter, update, or insert data.
- pyODBC proves invaluable for tasks ranging from querying and reporting to developing sophisticated data-driven applications.
Enroll in our Python programming course in affiliation with the best in the industry and upskill!
How to Install pyODBC?

Installing pyODBC is a straightforward process that involves a few simple steps. Here we will enlighten you with a step-by-step guide to help you get pyODBC up and running on your system.
Before installing pyODBC, ensure you have Python installed on your system. You can visit Python’s official website to download and install the updated version of Python.
pyODBC relies on the ODBC driver manager and database-specific drivers to establish database connections. Depending on your database systems, like MS SQL Server, MySQL, and PostgreSQL, you need to install the corresponding ODBC driver and any additional dependencies required by your database.
The installation for Windows, macOS X, and Linux is similar. One single command with the prerequisites mentioned previously and their default terminals.
Run the following command in your default terminal (CMD for Windows, Homebrew for macOS X, and Linux’s terminal):
pip install pyodbc
Run the following command to check if your installation was successful:
import pyodbc
Do you want to master computer programming using Python? Check out our Python Tutorial: For Beginners.
pyODBC Syntax and Examples: 10 Essential Code Snippets
As databases are crucial to many applications, understanding pyODBC’s syntax is necessary for data interactions.
The latest syntax of pyODBC contains functions for creating connections, cursors, executing SQL commands, and handling results. It’s essential to refer to the official documentation or trusted sources to keep abreast of updates or changes.
Connecting to a Database: Run this query to connect with the database.
import pyodbc conn = pyodbc.connect('DRIVER={driver_name}; SERVER=server_name; DATABASE=database_name; UID=user_id; PWD=password')
Fetching Database Drivers: To list available ODBC drivers:
drivers = [driver for driver in pyodbc.drivers()]
print(drivers)
Executing SQL Queries: After establishing a connection, SQL queries can be executed.
cursor = conn.cursor()
cursor.execute("SELECT * FROM table_name")
Fetching Results: Retrieve all results or just one.
rows = cursor.fetchall()
for row in rows:
print(row)
or,
row = cursor.fetchone()
print(row)
Parameterized Queries: For security, use parameterized queries.
cursor.execute("INSERT INTO table_name (column1, column2) VALUES (?, ?)", (value1, value2))
Updating Records: This query can be used to update the data stored in the table.
cursor.execute("UPDATE table_name SET column1 = ? WHERE column2 = ?", (value1, value2))
conn.commit()
Deleting Records: Using this command, you can delete an element from the table.
cursor.execute("DELETE FROM table_name WHERE column1 = ?", (value1,))
conn.commit()
Creating Tables: Use this command to create a table in pyODBC.
cursor.execute("CREATE TABLE new_table (column1 type1, column2 type2)")
Closing Connection: Post operations, always close the connection.
conn.close()
Are you interested in opting for development as your full-time career? Learn how to become a Python developer.
How to Link pyODBC with Various Databases?
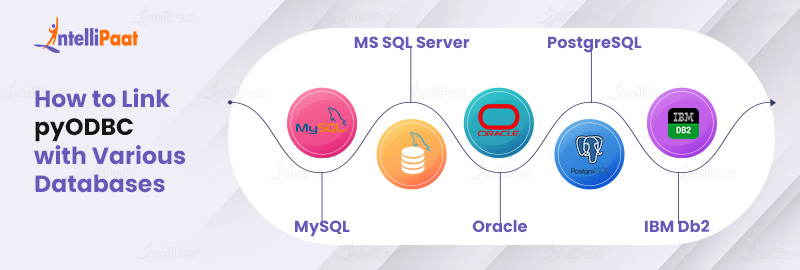
The downloading and installation process of pyODBC is the same for every database, as we have mentioned above. However, the integration with pyODBC differs. Here, we give you a step-by-step guide to link pyODBC with popular databases.
Linking pyODBC to MySQL
Step 1: Essential Preparations
- Ascertain that Python is duly installed on your system.
- Acquire and install the official MySQL ODBC driver for your operating system. This driver acts as the linchpin, connecting pyODBC to the MySQL environment.
Step 2: Library Installation
To establish the desired connectivity, first procure the requisite Python library:
pip install pyodbc
Step 3: Assembling Database Details
To ensure a seamless connection, specific information related to your MySQL database is paramount:
- Host (or IP Address)
- Database Name
- User Credentials (Username & Password)
Step 4: Constructing the Connection String
Draft a detailed connection string incorporating the data collated above. This structured string dictates to pyODBC the mode of interaction with MySQL.
connection_string = (
“DRIVER={MySQL ODBC 8.0 Unicode Driver};”
“SERVER=your_host;”
“DATABASE=your_db_name;”
“UID=your_username;”
“PWD=your_password;”
“OPTION=3;”
)
Note: The specific driver name, like “MySQL ODBC 8.0 Unicode Driver”, may differ depending on the driver’s version.
Step 5: Engaging the Connection
Use the connect function provided by pyODBC, coupled with the connection string, to initiate the connection.
import pyodbc
conn = pyodbc.connect(connection_string)
Step 6: Executing SQL Queries
Upon securing a connection, SQL commands can be executed with ease. For instance, here’s how to retrieve all entries from ‘example_table’.
cursor = conn.cursor()
cursor.execute("SELECT * FROM example_table")
for entry in cursor:
print(entry)
Step 7: Safely Terminating the Connection
Once all operations conclude, promptly close the connection to ensure optimal resource management.
cursor.close()
conn.close()
Linking pyODBC to MS SQL Server
Step 1: Acquire MS SQL ODBC Driver
Ensure that you have the ODBC driver for SQL Server installed on your system. Microsoft offers the ODBC Driver for SQL Server, which can be downloaded from its official website: Microsoft ODBC Driver for SQL Server.
Step 2: Database Connection String
Formulate a connection string that has the necessary details for establishing a connection, including the driver, server name, database name, and user credentials.
connection_string = (
'DRIVER={ODBC Driver 17 for SQL Server};'
'SERVER=your_server_name;'
'DATABASE=your_database_name;'
'UID=your_username;'
'PWD=your_password;'
)
Step 3: Establish a Connection
Use the formulated connection string to initiate a connection to your MS SQL Server database.
import pyodbc
conn = pyodbc.connect(connection_string)
Step 4: Execute SQL Commands
With the connection established, you can now use a cursor object to execute SQL commands. Start by creating a cursor, then execute a query.
cursor = conn.cursor()
cursor.execute('SELECT * FROM your_table_name')
Step 5: Fetch Results
Retrieve the results from your executed command. You can fetch a single row using fetchone() or all rows using fetchall().
rows = cursor.fetchall()
for row in rows:
print(row)
Step 6: Close the Connection
It’s imperative to close the database connection once your operations are complete to free up resources.
conn.close()
Learn the basics of SQL through our SQL Tutorial for absolute beginners.
Linking pyODBC to PostgreSQL
Step 1: Install PostgreSQL ODBC Driver
To interface with PostgreSQL via pyODBC, you need the PostgreSQL ODBC driver. Visit the official website and download the appropriate version for your operating system. After downloading, follow the installation instructions.
Step 2: Setup Data Source Name (DSN)
- On Windows:
- Navigate to Administrative Tools > ODBC Data Sources
- Switch to the ‘System DSN’ tab and click ‘Add’
- Select ‘PostgreSQL ANSI’ or ‘PostgreSQL Unicode’ and click ‘Finish’
- Fill in the required details, such as database name, server, port, user ID, and password. Click ‘Save’.
- On macOS/Linux:
- Edit or create an odbc.ini file in your home directory
- Add the following configuration:
[PostgreSQL]
Description=PostgreSQL connection
Driver=PostgreSQL Unicode
Database=your_database_name
Servername=your_server_address
Port=5432
UID=your_username
PWD=your_password
Step 3: Connect to PostgreSQL Using pyODBC
Utilize pyODBC to establish a connection with the PostgreSQL database using the defined DSN.
import pyodbc
connection = pyodbc.connect('DSN=PostgreSQL;UID=your_username;PWD=your_password')
cursor = connection.cursor()
Step 4: Execute Queries
With the connection established, you can now run SQL queries.
cursor.execute("SELECT * FROM your_table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
Step 5: Close the Connection
Ensure that after your operations are complete, you close the connection to free up resources.
connection.close()
Step 6: Handle Errors
Integrate error handling for enhanced resilience.
try:
connection = pyodbc.connect('DSN=PostgreSQL;UID=your_username;PWD=your_password')
except pyodbc.Error as ex:
print("Connection error:", ex)
Linking pyODBC to Oracle
Step 1: Install Oracle Client Libraries
Before connecting to Oracle with pyODBC, ensure Oracle Client Libraries are installed on your system. These libraries are crucial for the ODBC driver to interact with the Oracle database.
- Download the libraries from Oracle’s official site: Oracle Instant Client Downloads.
Step 2: Install ODBC Driver
Next, the Oracle ODBC driver, which bridges pyODBC and Oracle’s client libraries, needs installation.
- Download the appropriate ODBC driver from Oracle ODBC Downloads.
- Follow the installation instructions provided by Oracle.
Step 3: Set Environment Variables
After installation, add Oracle’s client library path to your system’s environment variables.
- For Windows: Add the path to PATH environment variable
- For Linux/Unix: Update the LD_LIBRARY_PATH with the client library’s path.
Step 4: Install pyODBC
Use pip, Python’s package installer, to install pyODBC.
pip install pyodbc
Step 5: Establish Connection
With dependencies in place, Python can now communicate with the Oracle database. Create a connection string and use pyODBC to establish the link.
import pyodbc
connection_string = (
"DRIVER={Oracle in OraClient11g_home1};"
"DBQ=your_service_name;"
"UID=your_username;"
"PWD=your_password"
)
connection = pyodbc.connect(connection_string)
Replace your_service_name, your_username, and your_password with your Oracle database details.
Step 6: Execute Queries
With the connection set, you can now execute SQL queries.
cursor = connection.cursor()
cursor.execute("SELECT * FROM your_table_name")
rows = cursor.fetchall()
for row in rows:
print(row)
Step 7: Close the Connection
Post-operation, always ensure that you close the database connection to free up resources.
connection.close()
Linking pyODBC to IBM Db2
Step 1: Prerequisites
- Download and install the IBM Db2 ODBC driver, which acts as the intermediary between pyODBC and the IBM Db2 database.
Step 2: Installation
Prior to executing any database connection, you must have the required Python libraries installed.
pip install pyodbc
Step 3: Database Configuration
For a successful connection, the following IBM Db2 database details are necessary:
- Hostname (or IP address)
- Database Name
- Port Number (default for Db2 is usually 50000)
- Username
- Password
Step 4: Formulating the Connection String
Create a connection string encapsulating the details mentioned. This string is pivotal, instructing pyODBC on the mode of interaction with the IBM Db2 database.
connection_string = (
"DRIVER={IBM DB2 ODBC DRIVER};"
"DATABASE=your_database_name;"
"HOSTNAME=your_hostname;"
"PORT=your_port_number;"
"PROTOCOL=TCPIP;"
"UID=your_username;"
"PWD=your_password;"
)
Note: Ensure the driver name in the connection string corresponds precisely to the name registered during the IBM Db2 ODBC driver installation.
Step 5: Establishing the Connection
Use pyODBC’s connect method, integrating the connection string, to initiate the connection.
import pyodbc
connection = pyodbc.connect(connection_string)
Step 6: Implementing SQL Commands
With the connection in place, SQL commands can now be executed. Below is a sample of extracting data from a table titled ‘example_table’.
cursor = connection.cursor()
cursor.execute("SELECT * FROM example_table")
for row in cursor:
print(row)
Step 7: Terminate the Connection
Once your operations conclude, responsibly close the connection to optimize resource usage.
cursor.close()
connection.close()
Brush up on your MySQL concepts for your next interview with our MySQL interview questions.
Career Transition
Troubleshooting pyODBC Errors
Addressing pyODBC errors requires a methodical approach. By familiarizing yourself with common pitfalls, you can ensure smoother database operations and efficient troubleshooting.
Let’s explore the ten most common errors and their resolutions:
Driver Not Found:
Error: ‘IM002’, ‘[IM002] [Microsoft][ODBC Driver Manager] Data source name not found and no default driver specified’
Solution: Ensure the ODBC driver for your database is installed. For instance, for PostgreSQL, you would need the PostgreSQL ODBC driver. Verify that the driver’s name in your connection string matches the installed driver’s name.
connection = pyodbc.connect('DRIVER={ODBC Driver for PostgreSQL};...')
Invalid Connection String:
Error: Errors related to invalid DSNs or credentials.
Solution: Double-check your connection string. Ensure all parameters (e.g., server name, database name) are correctly specified.
Database Not Found:
Error: Database ‘XYZ’ does not exist.
Solution: Confirm that the database name is correct and that the database exists on the server.
Table Not Found:
Error: ’42S02′, ‘[42S02] [Microsoft][ODBC SQL Server Driver][SQL Server]Invalid object name’
Solution: Ensure the table name is correct and exists in the database. Also, verify that you’re connected to the correct database.
Column Not Found:
Error: Column ‘ColumnName’ does not belong to table ‘Table.’
Solution: Double-check your SQL query and the table schema. Ensure the column names in your query match the actual column names in the table.
Permission Denied:
Error: Permission denied for relation XYZ
Solution: Ensure the user credentials used in the connection string have appropriate permissions for the operation you’re trying to perform.
Timeout Error:
Error: Query timeout expired
Solution: This error signifies a long-running query. Optimize your query or increase the timeout duration in the connection string.
connection = pyodbc.connect('DRIVER={...};TIMEOUT=30;...')
Data Type Mismatch:
Error: Disallowed implicit conversion from data type XYZ to data type ABC
Solution: Ensure that the data you’re trying to insert or update matches the column’s data type in the database.
Incorrect Cursor Usage:
Error: No results. Previous SQL was not a query.
Solution: Ensure you’re using the appropriate cursor methods. For instance, use cursor.execute() for queries that don’t return results and cursor.fetchall() for those that do.
Not Closing Connections:
Error: Excessive connections or resource exhaustion
Solution: Always close your connection after operations to free up resources. Implement the below statement to ensure connections close even if errors occur:
with pyodbc.connect('DRIVER={...}') as connection:
# Operations here
Are you curious to learn more about MongoDB and its offerings? Check out our Mongo DB Tutorial.
Best Practices in pyODBC to Run Queries Faster
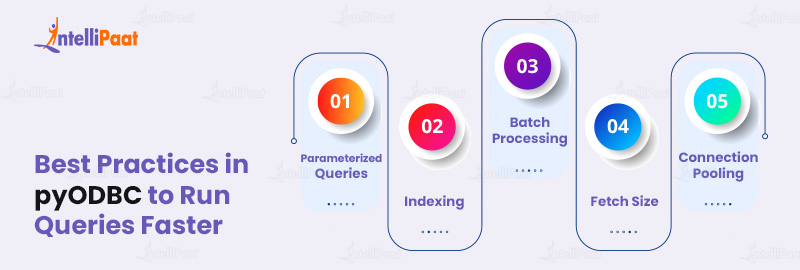
To improve how quickly queries are executed using pyODBC, let’s go over the important things to do. This will help you work well with different database systems.
Parameterized Queries: Parameterized queries optimize query execution by preparing statements in advance. This reduces the parsing time for repetitive queries. Utilize parameterized queries to prevent SQL injection and improve performance.
import pyodbc
conn = pyodbc.connect('your_connection_string')
cursor = conn.cursor()
query = "SELECT * FROM orders WHERE customer_id = ?"
cursor.execute(query, (customer_id,))
Indexing: Apply proper indexing to speed up query processing. Indexes facilitate quicker data retrieval by creating a structured path through the database. Identify the frequently used columns in WHERE, JOIN, and ORDER BY clauses for indexing.
Batch Processing: Use batch processing for multiple INSERT, UPDATE, or DELETE operations. It reduces overhead by minimizing round-trips between the application and the database. Example:
data = [(value1,), (value2,), ...]
query = "INSERT INTO table_name (column_name) VALUES (?)"
cursor.executemany(query, data)
Fetch Size: Optimize the fetch size to balance memory and retrieval speed when fetching big result sets. Adjust the fetchmany method’s argument to control the number of rows retrieved per fetch.
cursor.execute("SELECT * FROM large_table")rows = cursor.fetchmany(size=1000) # Fetches 1000 rows at a time
Connection Pooling: Implement connection pooling to reuse established database connections. Pooling improves performance by reducing the need to create new connections for every query.
import pyodbc
from sqlalchemy import create_engine
connection_string = 'your_connection_string'
engine = create_engine(connection_string, pool_size=10, max_overflow=20)
Check out our blog on SQL Vs. Python to learn more about their differences in depth.
Advantages of Using pyODBC
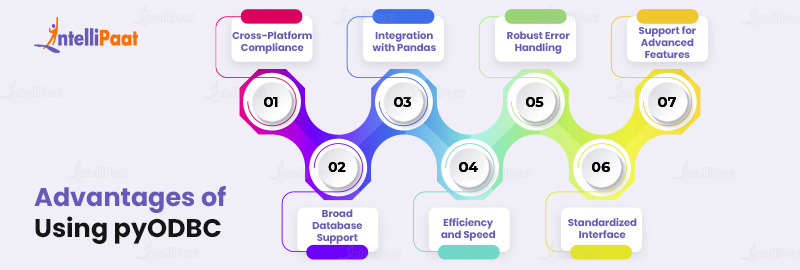
Here are the factors for which pyODBC gives you the upper hand over other pyODBC alternatives:
- Cross-Platform Compliance: pyODBC is platform-agnostic. This Python module works well on Windows, Linux, and macOS X computers. You can create an app on macOS X and use it on a Linux server without changing anything.
- Broad Database Support: pyODBC can work with many different databases as long as there is an ODBC driver available. No matter which database you’re using—MySQL, Microsoft SQL Server, or Oracle—pyODBC can help you.
Example: connection = pyodbc.connect(‘DRIVER={SQL Server};SERVER=server_name;DATABASE=database_name;UID=user_name;PWD=password’).
- Integration with Pandas: Pandas make it easier to manipulate and analyze data. You can easily use pyODBC with Pandas to convert database data into a DataFrame.
Example: df = pd.read_sql_query(‘SELECT * FROM table_name’, connection).
- Efficiency and Speed: pyODBC uses the ODBC API, which makes it fast and efficient when running queries and getting results. It translates to faster data extraction and minimal latency in real-time applications.
- Robust Error Handling: It provides helpful error messages for fixing connection issues or SQL errors. This feedback generation can be valuable during development, guiding the developer to the issue’s root.
- Standardized Interface: pyODBC uses the ODBC standard for a consistent API, making it easier for developers to learn. In case you’re switching databases or working on diverse projects, the interaction remains uniform.
- Support for Advanced Features: pyODBC goes beyond basic CRUD operations. It also supports stored procedures, parameterized queries, and multiple result sets.
For example, you can call a stored procedure using: cursor.execute(“{CALL stored_procedure_name}”).
Strengthen your SQL concepts through our SQL Interview Questions and land your dream job.
Courses you may like
What are the Alternatives to pyODBC?
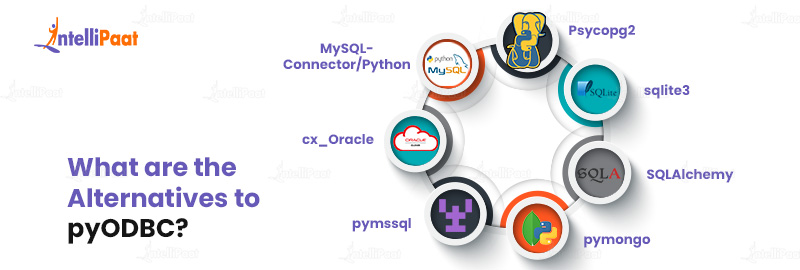
Here, we present you the top alternatives to pyODBC for connecting your databases:
- sqlite3: Part of Python’s standard library, sqlite3 caters specifically to SQLite databases, offering a lightweight, disk-based database without necessitating a separate server process. Its integration as a built-in Python module assures stability and consistent updates.
- SQLAlchemy: An expansive SQL toolkit and Object-Relational Mapping (ORM) system, SQLAlchemy abstracts the differences between various types of databases, facilitating a more Pythonic interaction with databases. Its advanced features, including connection pooling, elevate its utility beyond mere database connections.
- Psycopg2: Tailored for PostgreSQL, Psycopg2 stands out as an efficient Python adapter for this database system. Its reputation for functionality and reliability makes it a go-to choice for PostgreSQL-focused applications.
- MySQL-Connector/Python: As MySQL’s endorsed Python driver, MySQL-Connector ensures native connections to MySQL databases, eliminating dependencies on extra libraries or tools. This pure Python solution emphasizes seamless connectivity.
- cx_Oracle: This Python extension facilitates connections to Oracle databases. Following the Python database API specification, cx_Oracle emerges as a dependable tool for Oracle database operations.
- pymssql: Catering to Microsoft SQL Server, pymssql presents a straightforward database interface for Python, aligning with the DB-API 2.0 specification. Its ease of use and specificity make it ideal for SQL Server operations.
- pymongo: For developers engaged with MongoDB, pymongo, the official MongoDB driver for Python, serves as a valuable resource. It simplifies interactions and manipulations with MongoDB collections and documents.
Enhance your interview preparations with us. Check out our well-curated list of Top Python Interview Questions.
Conclusion
The blog provides you with a complete understanding of pyODBC, guiding readers through its installation and practical applications. By following the installation steps and learning its usage, readers can now confidently interact with various databases using Python. To further deepen your knowledge, consider exploring advanced topics like optimizing query performance, utilizing stored procedures, and handling transactions.
Despite the emergence of alternative libraries, pyODBC remains a favored choice for developers for several reasons. Its extensive support for different database systems, cross-platform compatibility, and consistent API structure contribute to its enduring popularity. PyODBC is reliable for developers, whether they are new or experienced. As technology changes, pyODBC remains a valuable tool for developers due to its versatility and track record.
Resolve your doubts and catch up with your fellow learners. Join Intellipaat’s community of Python coders.
The post What is Pyodbc? Installation to Implementation appeared first on Intellipaat Blog.
Blog: Intellipaat - Blog
Leave a Comment
You must be logged in to post a comment.