What is a Palindrome Number? (in C, Java, and Python)
Palindrome numbers, a fascinating concept in mathematics and computer science, exhibit a remarkable property: they remain the same when reading forward and backward. By understanding the logic behind palindrome number identification and employing programming techniques, you can sharpen your programming skills and gain insight.
Table of Contents
- What is a Palindrome Number?
- Importance and Applications of Palindrome Numbers
- Palindrome Number in C
- Palindrome Number in Java
- Palindrome Number in Python
- Conclusion
Learn Data Structures and Algorithms in this comprehensive video course:
{
“@context”: “https://schema.org”,
“@type”: “VideoObject”,
“name”: “Data Structures And Algorithms | DSA Course | Data Structures And Algorithms Tutorial | Intellipaat”,
“description”: “What is a Palindrome Number? (in C, Java, and Python)”,
“thumbnailUrl”: “https://img.youtube.com/vi/3Aid2c1QafI/hqdefault.jpg”,
“uploadDate”: “2023-07-21T08:00:00+08:00”,
“publisher”: {
“@type”: “Organization”,
“name”: “Intellipaat Software Solutions Pvt Ltd”,
“logo”: {
“@type”: “ImageObject”,
“url”: “https://intellipaat.com/blog/wp-content/themes/intellipaat-blog-new/images/logo.png”,
“width”: 124,
“height”: 43
}
},
“embedUrl”: “https://www.youtube.com/embed/3Aid2c1QafI”
}
What is a Palindrome Number?
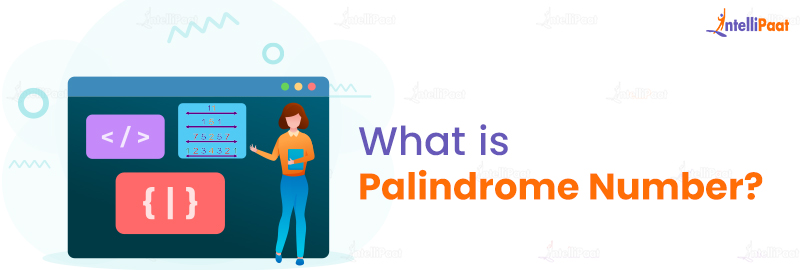
When a number is read both forward and backward, it is said to be a palindrome. Palindrome numerals include, for instance, 121, 2332, and 789987. In C, you can check for a palindrome by converting the number to a string and comparing the characters from both ends. Using a loop, check if the characters match until the middle of the string is reached.
In Java, the process is similar. Convert the number to a string and use two pointers, one at the beginning and the other at the end, to compare the characters.
In Python, convert the number to a string, reverse it using string slicing, and compare it with the original string. If both are the same, it’s a palindrome number.
Importance and Applications of Palindrome Numbers
Palindrome numbers hold significance and find applications in various fields, including computer programming. Let’s explore their importance and applications.
- Algorithmic Programming:
- Palindrome number algorithms are excellent exercises for developing logical thinking and problem-solving skills in programming languages like C, Java, and Python.
- Implementing palindrome number checks can help programmers enhance their understanding of loops, conditionals, and string manipulation.
- User Input Validation:
- Palindrome number checks can be used in user input validation scenarios. For example, when users must enter a numeric code or identifier, validating whether the input is a palindrome number can ensure data integrity and accuracy.
- Programmers can implement input validation checks in C, Java, or Python to verify whether the provided input is a palindrome number.
- String Manipulation:
- Palindrome numbers are closely related to palindromic strings. The algorithms that check palindrome numbers can be extended to validate palindromic strings.
- In all three programming languages (C, Java, and Python), developers can apply similar techniques to check if a given string is a palindrome by comparing characters from both ends of the string.
- Error Detection and Data Integrity:
- Palindrome numbers can be used as a simple error detection mechanism in various applications and protocols, such as network communication or checksum calculations.
- Developers can utilize palindrome number checks in C, Java, or Python to identify accidental alterations or errors in transmitted or stored data, ensuring data integrity and reliability.
- Recreational Puzzles:
- Developers can create fun programming challenges or puzzles based on palindrome numbers in C, Java, or Python. This provides an engaging and entertaining way to explore numerical patterns and mathematical interests.
Palindrome Number in C
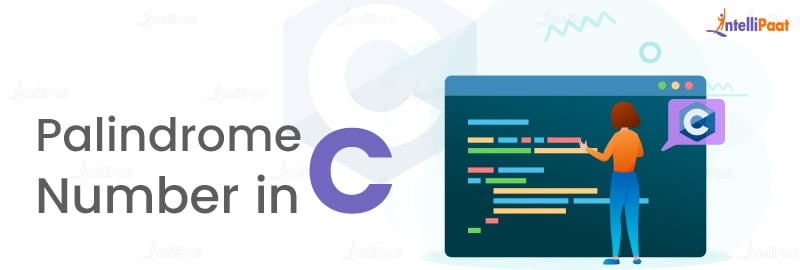
Implementation in C
To determine if a number is a palindrome in the C programming language, you can follow these steps:
- Take the input number from the user.
- Create a copy of the input number for later comparison.
- Initialize variables to store the reverse and remainder of the number.
- Use a while loop to reverse the number.
- In each iteration, extract the number’s last digit using the modulo operator (%).
- Multiply the reverse by 10 and add the extracted digit.
- Update the number by dividing it by 10.
- Repeat steps 5-7 until the number becomes 0.
- Compare the reversed number with the copy of the input number.
- If they are equal, the number is a palindrome. Otherwise, it is not.
Whether you’re a beginner or an experienced developer, this C Tutorial has something for you!
Step-by-Step Explanation
- Ask the user to input a number.
- Create a copy of the input number and store it in a separate variable.
- Initialize variables for storing the reverse (initially set to 0) and the remainder (initially set to 0).
- Start a while loop that continues until the input number becomes 0.
- Inside the loop, extract the last digit of the input number using the modulo operator (%).
- Multiply the reverse by 10 and add the extracted digit to reverse the number.
- Update the input number by dividing it by 10 to remove the last digit.
- Repeat steps 5-7 until the input number becomes 0.
- Compare the reversed number with the copy of the input number.
- If they are equal, print a message indicating that the number is a palindrome. Otherwise, print a message indicating that the number is not a palindrome.
Sample Code and Output
The code provided below is a basic implementation of palindrome number testing in C.
Code:
#include
int main() {
int number, copy, reverse = 0, remainder;
printf("Enter a number: ");
scanf("%d", &number);
copy = number;
while (number != 0) {
remainder = number % 10;
reverse = reverse * 10 + remainder;
number /= 10;
}
if (copy == reverse) {
printf("%d is a palindrome number.n", copy);
} else {
printf("%d is not a palindrome number.n", copy);
}
return 0;
}
Sample Output 1:
Enter a number: 12321
12321 is a palindrome number.
Sample Output 2:
Enter a number: 12345
12345 is not a palindrome number.
Palindrome Number in Java
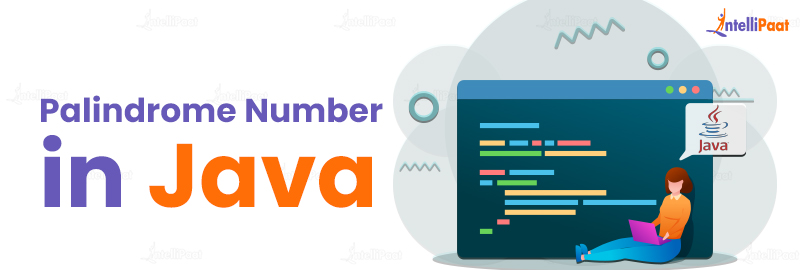
Implementation in Java
To check whether a number is a palindrome in Java, you can follow these steps:
- Take the input number from the user.
- Create a copy of the input number for later comparison.
- Convert the input number to a string for easier manipulation.
- Use two pointers, one starting from the string’s beginning and the other from the end.
- Compare the characters at the two pointers.
- Move the left pointer to the right and the right pointer to the left.
- Repeat steps 5-6 until the pointers meet or cross each other.
- If all the characters match, the number is a palindrome. Otherwise, it is not.
Step-by-Step Explanation
- Ask the user to input a number.
- Create a copy of the input number and store it in a separate variable.
- Convert the input number to a string using the Integer.toString() method.
- Initialize two pointers: left, starting from index 0, and right, starting from the last index of the string.
- Start a while loop that continues until the left pointer is less than or equal to the right pointer.
- Inside the loop, compare the characters at the left and right indices using the charAt() method.
- If the characters are not equal, print a message indicating that the number is not a palindrome and exit the loop.
- Move the left pointer to the right by incrementing its value and move the right pointer to the left by decrementing its value.
- Repeat steps 6-8 until the left pointer is less than or equal to the right pointer.
- If the loop completes without finding non-matching characters, print a message indicating that the number is a palindrome.
This Java Tutorial will teach you everything you need to know to get started with Java!
Sample Code and Output
The above code is a basic implementation of palindrome number testing in Java.
Code:
import java.util.Scanner;
public class PalindromeNumber {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int number = scanner.nextInt();
int copy = number;
String numberString = Integer.toString(number);
int left = 0;
int right = numberString.length() - 1;
while (left <= right) {
if (numberString.charAt(left) != numberString.charAt(right)) {
System.out.println(copy + " is not a palindrome number.");
return;
}
left++;
right--;
}
System.out.println(copy + " is a palindrome number.");
}
}
Sample Output 1:
Enter a number: 12321
12321 is a palindrome number.
Sample Output 2:
Enter a number: 12345
12345 is not a palindrome number.
Career Transition
Palindrome Number in Python
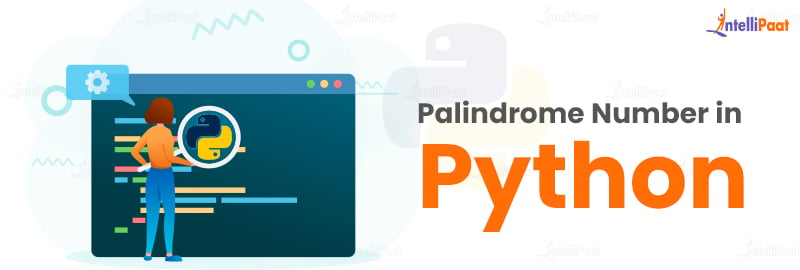
Implementation in Python
Follow these steps to check whether a number is a palindrome in Python:
- Take the input number from the user.
- Create a copy of the input number for later comparison.
- Convert the input number to a string for easier manipulation.
- Use string slicing to reverse the string representation of the number.
- Compare the reversed string with the original string.
- If they are equal, the number is a palindrome. Otherwise, it is not.
Step-by-Step Explanation
- Ask the user to input a number.
- Create a copy of the input number and store it in a separate variable.
- Convert the input number to a string using the str() function.
- Use string slicing with a step of -1 to reverse the string representation of the number.
- Compare the reversed string with the original string.
- If they are equal, print a message indicating that the number is a palindrome. Otherwise, print a message indicating that the number is not a palindrome.
Whether you’re a beginner or an experienced developer, this Python course has something for you!
Sample Code and Output
The code provided above is a basic implementation of palindrome number testing in Python.
Code:
number = int(input("Enter a number: "))
copy = number
number_string = str(number)
reversed_string = number_string[::-1]
if number_string == reversed_string:
print(copy, "is a palindrome number.")
else:
print(copy, "is not a palindrome number.")
Sample Output 1:
Enter a number: 12321
12321 is a palindrome number.
Sample Output 2:
Enter a number: 12345
12345 is not a palindrome number.
Click here to access our Python Interview Questions and start practicing today!
Conclusion
In conclusion, palindrome numerals retain considerable significance and have a wide range of uses due to their special trait of remaining the same when read both forward and backward. We have looked at the significance of palindrome numbers in the context of computer programming and error detection. Additionally, we have delved into their implementation in three popular programming languages: C, Java, and Python. By providing step-by-step explanations, sample codes, and outputs, we have equipped you with the necessary knowledge to identify and work with palindrome numbers in each language.
The post What is a Palindrome Number? (in C, Java, and Python) appeared first on Intellipaat Blog.
Blog: Intellipaat - Blog
Leave a Comment
You must be logged in to post a comment.