React Carousel – Explained
Before diving into React Carousel, there are certain prerequisites that developers should be familiar with. Firstly, a solid understanding of React and JavaScript is essential, as React Carousel heavily relies on these technologies. Familiarity with React components, state management, and event handling is crucial. Additionally, proficiency in CSS is important for styling and customizing the carousel. Prior knowledge of responsive web design principles and media queries is beneficial for creating a responsive carousel that adapts to different screen sizes.
Table of Contents:
- What is React Carousel?
- Different Types of React Carousels
- Best Practices for Using React Carousels
- Benefits of React Carousel
- What are the Use Cases of React Carousel?
- Conclusion
Check out our Full Stack Web Developer Course on YouTube:
{
“@context”: “https://schema.org”,
“@type”: “VideoObject”,
“name”: “Full Stack Web Development Course 2023 | Full Stack Developer Course | Intellipaat”,
“description”: “React Carousel – Explained”,
“thumbnailUrl”: “https://img.youtube.com/vi/b92T8aCOwS0/hqdefault.jpg”,
“uploadDate”: “2023-07-21T08:00:00+08:00”,
“publisher”: {
“@type”: “Organization”,
“name”: “Intellipaat Software Solutions Pvt Ltd”,
“logo”: {
“@type”: “ImageObject”,
“url”: “https://intellipaat.com/blog/wp-content/themes/intellipaat-blog-new/images/logo.png”,
“width”: 124,
“height”: 43
}
},
“embedUrl”: “https://www.youtube.com/embed/b92T8aCOwS0”
}
What is React Carousel?
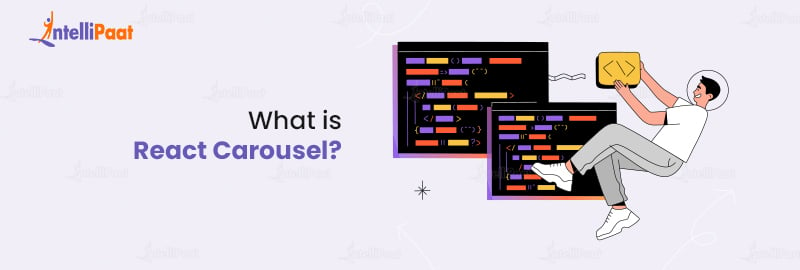
A React carousel is a component used in user interfaces to present a collection of images, videos, or other content in a visually appealing slideshow format. It offers an interactive way to showcase multiple items within a limited space, such as a website or application.
Typically, a React carousel includes a container that holds individual slides. Users can navigate through the slides by interacting with navigation buttons, using swipe gestures on touch-enabled devices, or by enabling automatic transitions between slides at a specified interval.
To create React carousels, developers leverage the React JavaScript library, which is widely adopted for building user interfaces. Various React carousel libraries are available, offering pre-built components and functionalities that simplify the integration of carousels into React applications.
These libraries provide features like responsive layouts that adapt to different screen sizes, customizable transition effects, autoplay options, and navigation controls, enhancing the versatility and user experience of the carousels.
Implementation Example:
To showcase the utilization of React Carousel, let us examine a straightforward image slider. Initially, it is imperative to install the necessary packages.
npm install react-responsive-carousel
Next, import the necessary components and define the carousel structure as shown below:
import { Carousel } from 'react-responsive-carousel'; import 'react-responsive-carousel/lib/styles/carousel.min.css'; const MyCarousel = () => { return (![]()
Image 1 Description
![]()
Image 2 Description
); }![]()
Image 3 Description
In this example, we import the Carousel component from the ‘react-responsive-carousel’ package. We also import the default CSS styles for the carousel. Inside the component, we define the carousel structure by wrapping each slide’s content (image and description) in a
To learn more about React JS check out Intellipaat’s React Certification course!
Different Types of React Carousels
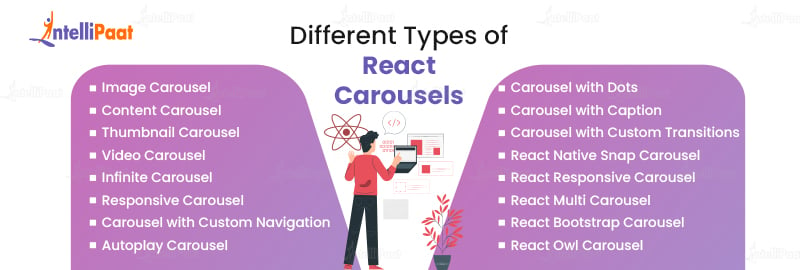
Here are the distinct types of React carousels, highlighting their unique features and benefits and providing code examples to facilitate implementation:
Image Carousel
An image carousel represents the prevalent form of carousel utilized for displaying a series of images or visual content in a smooth and uninterrupted manner. It empowers users to effortlessly navigate through the images via navigation arrows or pagination controls.
import React from 'react'; import Carousel from 'react-responsive-carousel'; const ImageCarousel = () => { return (![]()
); };![]()
Content Carousel
Content carousels are ideal for displaying textual content such as testimonials or product descriptions. They provide a way to present information in a compact and visually appealing manner. Here’s an example:
import React from 'react'; import Carousel from 'react-responsive-carousel'; const ContentCarousel = () => { return (Testimonial 1
Lorem ipsum dolor sit amet, consectetur adipiscing elit.
Testimonial 2
Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris.
); };Testimonial 3
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore.
Thumbnail Carousel
Thumbnail carousels provide a visual preview of multiple images or content items. Users can navigate through the main carousel while simultaneously viewing the thumbnails. Here’s an example:
import React from 'react'; import Carousel from 'react-responsive-carousel'; const ThumbnailCarousel = () => { return (![]()
Description 1
![]()
Description 2
); };![]()
Description 3
Video Carousel
Video carousels allow the integration of videos within a carousel component. This type of carousel is perfect for showcasing video content in a visually appealing manner.
import React from 'react'; import Carousel from 'react-responsive-carousel'; const VideoCarousel = () => { return (); };
Infinite Carousel
Infinite carousels allow continuous looping of content items, providing a seamless user experience. When the carousel reaches the last item, it seamlessly transitions back to the first item. Here’s an example:
import React from 'react'; import Carousel from 'react-responsive-carousel'; const InfiniteCarousel = () => { return ({/* Content 1 */}{/* Content 2 */}{/* Content 3 */}); };
Responsive Carousel
Responsive carousels adapt to different screen sizes and orientations, ensuring optimal viewing on various devices. They dynamically adjust the number of visible items based on the available space. Here’s an example:
import React from 'react'; import Carousel from 'react-responsive-carousel'; const ResponsiveCarousel = () => { return ({/* Content 1 */}{/* Content 2 */}{/* Content 3 */}); };
Carousel with Custom Navigation
Carousels with custom navigation allow developers to create unique navigation controls such as buttons, thumbnails, or any other custom UI elements. Here’s an example using custom buttons:
import React from 'react'; import Carousel from 'react-responsive-carousel'; const CustomNavigationCarousel = () => { return ( ( )} renderArrowNext={(onClickHandler, hasNext, label) => ( )}> {/* Content items */} ); };
Autoplay Carousel
Autoplay carousels automatically scroll through the items without user interaction. This feature is commonly used to showcase featured products or promotional content. The following code example demonstrates an autoplay carousel using the React Carousel component:
import React from 'react'; import Carousel from 'react-carousel'; const AutoplayCarousel = () => { const items = ['item1', 'item2', 'item3']; return ( {items.map((item, index) => ({item}))} ); };
Carousel with Dots
Dotted navigation indicators are commonly used in carousels to show the current position and allow direct navigation to specific items. Here’s an example using the popular react-responsive-carousel library:
import React from 'react'; import { Carousel } from 'react-responsive-carousel'; import 'react-responsive-carousel/lib/styles/carousel.min.css'; const CarouselWithDots = () => { return (![]()
); }; export default CarouselWithDots;![]()
Carousel with Caption
Carousels with captions or legends provide additional context or descriptions for each item. Here’s an example using the popular react-responsive-carousel library:
import React from 'react'; import { Carousel } from 'react-responsive-carousel'; import 'react-responsive-carousel/lib/styles/carousel.min.css'; const CarouselWithCaption = () => { return (![]()
Caption 1
![]()
Caption 2
); }; export default CarouselWithCaption;![]()
Caption 3
Carousel with Custom Transitions
Custom transition effects can add visual appeal and uniqueness to your carousels. They can be achieved by utilizing CSS animations or third-party libraries. Here’s an example using the popular react-transition-group library:
import React from 'react'; import { CSSTransition, TransitionGroup } from 'react-transition-group'; import './CustomCarousel.css'; const CarouselWithCustomTransitions = () => { const items = ['Item 1', 'Item 2', 'Item 3']; return ( {items.map((item) => ({item}))} ); }; export default CarouselWithCustomTransitions;
React Native Snap Carousel
React Native Snap Carousel is a powerful carousel library specifically designed for React Native applications. It provides a smooth and performant carousel experience with support for touch gestures and swipe-based navigation. It also offers features like autoplay, pagination, and custom animations, making it an ideal choice for building mobile apps. Mentioned below is an example where the React Native Snap Carousel is used:
import Carousel from 'react-native-snap-carousel'; const MyCarousel = () => { const data = [/* Array of carousel items */]; return ( ( {/* Render carousel item */} )} /* Other carousel properties */ /> ); };
React Responsive Carousel
React Responsive Carousel is a versatile library that adapts to different types of screen sizes and device orientations. It automatically adjusts the number of visible items based on the available space, ensuring a responsive and user-friendly experience. This carousel also supports touch and mouse interactions, along with customizable transition effects. Mentioned below is an example using the React Responsive Carousel:
import Carousel from 'react-responsive-carousel'; const MyCarousel = () => { return ({/* Content of first carousel item */}{/* Content of second carousel item */}{/* Add more carousel items */} ); };
React Multi Carousel
React Multi Carousel is a feature-rich library that allows you to create multiple carousels on a single page with different configurations. It provides extensive customization options, including the ability to define breakpoints for responsive behavior, specify the number of visible items, and enable infinite looping. It also supports various navigation controls and autoplay functionality. Mentioned below is an example using React Multi Carousel:
import { CarouselProvider, Slider, Slide } from 'react-multi-carousel'; const MyCarousel = () => { return ( {/* Content of first carousel item */} {/* Content of second carousel item */} {/* Add more carousel items */} {/* Add navigation controls */} ); };
React Bootstrap Carousel
React Bootstrap Carousel is a carousel component that integrates seamlessly with the popular Bootstrap framework. It offers a range of features, such as swipe and keyboard navigation, autoplay, indicators, and carousel controls. Leveraging the power of Bootstrap, this carousel provides a clean and responsive design suitable for various web projects. Here’s an example that uses the React Bootstrap Carousel:
import { Carousel } from 'react-bootstrap'; const MyCarousel = () => { return ( {/* Content of first carousel item */} {/* Content of second carousel item */} {/* Add more carousel items */} ); };
React Owl Carousel
React Owl Carousel, a highly customizable carousel library, offers a wide range of options for creating visually appealing carousels. It supports numerous features, including responsive layouts, touch events, lazy loading, and autoplay, among others. Its vast array of configuration settings provides developers with great flexibility when it comes to customizing the carousel’s behavior and appearance. Mentioned below is an example that uses the React Owl Carousel:
import OwlCarousel from 'react-owl-carousel'; const MyCarousel = () => { return ({/* Content of first carousel item */}{/* Content of second carousel item */}{/* Add more carousel items */} ); };
To get in-depth knowledge of Reactjs check out our ReactJS Tutorial!
Career Transition
Best Practices for Using React Carousels
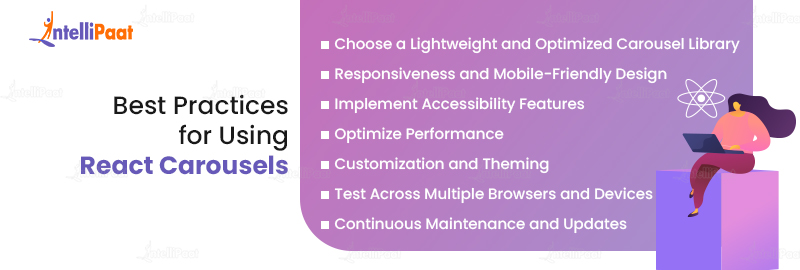
React carousels provide an interactive and visually appealing way to showcase content. However, using carousels effectively requires adhering to certain best practices to ensure optimal performance, accessibility, and user experience.
- Choose a Lightweight and Optimized Carousel Library: When selecting a React carousel library, prioritize lightweight options that are well-maintained and optimized for performance. Opting for a lightweight library ensures faster loading times, reduces unnecessary bloat, and improves the overall user experience. Additionally, regularly updated libraries provide bug fixes and compatibility enhancements, ensuring long-term support and stability.
- Responsiveness and Mobile-Friendly Design: In today’s mobile-centric world, ensuring that your React carousel is fully responsive and mobile-friendly is crucial. Use responsive design techniques to adapt the carousel’s layout and functionality to different screen sizes. Employ CSS media queries and React’s responsive components to achieve a seamless experience across various devices, enabling users to interact with the carousel effortlessly.
- Implement Accessibility Features: Accessibility should always be a top priority when developing web applications. Enhance the usability of your React carousel by implementing accessibility features such as keyboard navigation, focus management, and ARIA attributes. It ensures that users with disabilities or those who rely on assistive technologies can fully engage with and navigate the carousel’s content.
- Optimize Performance: To deliver a smooth and performant carousel experience, it is crucial to optimize its performance. Minimize unnecessary rendering by utilizing React’s lifecycle methods effectively. Employ lazy loading techniques to load images and content as needed, reducing initial load times. Implement code splitting and bundle optimization strategies to reduce the overall bundle size, resulting in faster page loads.
- Customization and Theming: Offering customization options and theming capabilities allows developers to adapt the carousel to match the project’s visual design and branding. Choose a carousel library that supports custom styling and theming, enabling you to easily modify the carousel’s appearance, transitions, and animations. This flexibility ensures seamless integration with your application design and improves the user experience.
- Test Across Multiple Browsers and Devices: Perform comprehensive testing across different browsers and devices to ensure cross-compatibility and consistent functionality. Various browsers and platforms may interpret code differently, leading to unexpected behavior. Utilize browser testing tools, such as Selenium or Puppeteer, and employ responsive design testing frameworks to verify that your React carousel functions flawlessly across various environments.
- Continuous Maintenance and Updates: Regularly update your React carousel implementation to address potential issues and security vulnerabilities. Keep up with the latest versions of React and your chosen carousel library to leverage performance improvements and bug fixes. Additionally, actively participate in the community around the carousel library to stay informed about new features, updates, and best practices shared by other developers.
Applying for a Front End Developer job? Read our blog on React JS Interview Questions and get yourself prepared!
Benefits of React Carousel
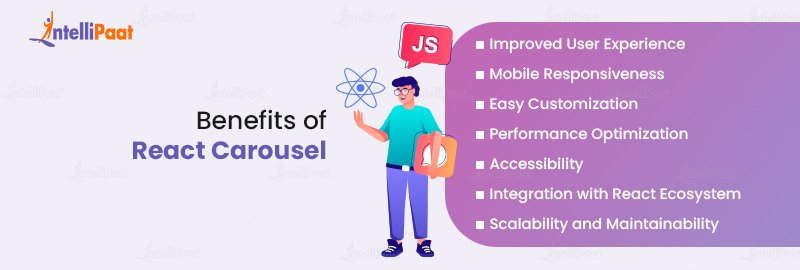
Let’s check out the top benefits of using React Carousel, highlighting its impact on user experience and website performance.
- Improved User Experience: React Carousel offers a visually appealing and interactive user experience. It allows for smooth transitions between slides, enabling users to effortlessly navigate through content such as product images, testimonials, or news articles. By enhancing user engagement, React Carousel contributes to a positive overall experience and helps retain visitors on a website for longer durations.
- Mobile Responsiveness: React Carousel enables developers to create carousels that adapt seamlessly to different screen sizes and orientations. With its responsive design capabilities, React Carousel ensures optimal viewing experiences across various devices, including smartphones, tablets, and desktops. This mobile-friendly approach improves accessibility and expands the reach of websites to a wider audience.
- Easy Customization: It offers developers a high level of flexibility and customization options. It provides a range of settings and properties that can be easily adjusted to meet specific design requirements. Developers can modify slide transition effects, navigation controls, autoplay settings, and more. This flexibility allows for the creation of unique and tailored carousels that align with the brand identity and aesthetics of a website.
- Performance Optimization: React Carousel is designed to optimize website performance. It leverages React’s virtual DOM (Document Object Model) and efficient rendering techniques to ensure smooth scrolling and transitions. By rendering only the necessary components when navigating through the carousel, React Carousel minimizes unnecessary resource consumption and maximizes performance, resulting in faster loading times and smoother user experiences.
- Accessibility: Accessibility is a very important facet of web development. React Carousel adheres to accessibility guidelines, which makes it simpler for users with disabilities to interact with the carousel content. By providing proper keyboard navigation support and semantic markup, React Carousel ensures that all users, including those who rely on assistive technologies, can explore and interact with the carousel effectively.
- Integration with the React Ecosystem: React Carousel seamlessly integrates with the wider React ecosystem, leveraging the benefits of popular React libraries and frameworks. It can be easily combined with other React components, such as modals, tabs, or accordions, to create more complex and interactive user interfaces. This integration simplifies the development process, enhances code reusability, and fosters a collaborative ecosystem for React developers.
- Scalability and Maintainability: As websites grow and evolve, scalability and maintainability become vital considerations. React Carousel’s modular structure and component-based approach contribute to code maintainability and scalability. Developers can reuse carousel components across different sections of a website, making updates and modifications easier to implement. This scalability ensures that the carousel remains manageable even as the website expands or undergoes design changes.
What are the Use Cases of React Carousel?
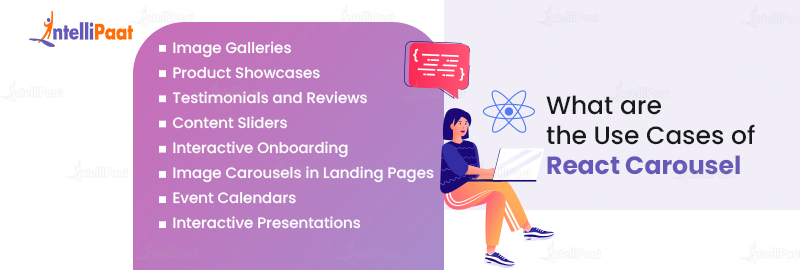
From image galleries to product showcases, React Carousel offers a range of possibilities for developers seeking to create a visually appealing and interactive web application. Let’s explore the use cases to know the implementation of react carousel in real life:
- Image Galleries: React Carousel is extensively employed to develop visually impressive image galleries. Its user-friendly navigation and seamless transitions enable users to move across through a curated collection of images. The carousel component offers customizable options to exhibit multiple images simultaneously or showcase a single image at a time, delivering an aesthetically captivating and engaging experience.
- Product Showcases: For e-commerce websites or applications, React Carousel enables developers to create interactive product showcases. By incorporating product images, descriptions, and additional details within the carousel, users can easily navigate through various offerings, enhancing the shopping experience and encouraging engagement.
- Testimonials and Reviews: React Carousel is an ideal choice for displaying testimonials and reviews on websites. By using carousel components, developers can showcase customer feedback in an engaging and interactive manner. Users can explore the different testimonials, ensuring that they are exposed to a range of opinions and experiences, thereby establishing trust and credibility.
- Content Sliders: React Carousel is a versatile tool for creating content sliders, enabling developers to present information in a concise manner that is visually appealing. Whether it’s highlighting key features, showcasing news articles, or displaying portfolio items, the carousel allows users to easily swipe or click through the content, ensuring a seamless browsing experience.
- Interactive Onboarding: React Carousel is commonly used for onboarding processes in web applications. By incorporating a carousel component, developers can guide users through a series of interactive screens, introducing key features, demonstrating functionality, and providing a smooth introduction to the application. This interactive onboarding experience enhances user engagement and facilitates user retention.
- Image Carousels in Landing Pages: Landing pages often employ image carousels to capture user’s attention and convey important messages. React Carousel offers a range of customization options, such as autoplay, navigation arrows, and indicators, enabling developers to create captivating and impactful landing page designs that effectively communicate their brand message.
- Event Calendars: React Carousel can be utilized to build event calendars with an intuitive and interactive interface. By representing each event as a carousel card, users can easily navigate through different dates, view event details, and even register or purchase tickets directly from the carousel. This use case provides a seamless user experience for event organizers and attendees.
- Interactive Presentations: React Carousel is an excellent tool for creating interactive presentations or slideshows. Developers can utilize the carousel component to incorporate various types of multimedia elements, such as videos, images, and interactive content, transforming traditional presentations into engaging experiences.
Courses you may like
Conclusion
In this blog, we have delved into the comprehensive exploration of React carousels, presenting an in-depth analysis of the ten most prominent types of React carousels. Each carousel type has been elucidated through the provision of code examples, ranging from fundamental carousels to image-based carousels, content-driven carousels, and video-focused carousels. Regardless of your specific requirements, there exists a carousel type tailored to fulfill your needs. Additionally, by integrating features such as autoplay, infinite scrolling, and personalized transitions, you can elevate the overall user experience to new heights.
Solve your queries related to the topic, visit our community, and catch up with other learners.
The post React Carousel – Explained appeared first on Intellipaat Blog.
Blog: Intellipaat - Blog
Leave a Comment
You must be logged in to post a comment.