Queue in Java: An Introduction with Example
This article will explain the java queue in detail and will cover its implementation and functions. Let’s discuss the agenda of the blog before directly jumping to the article.
- Java Queue
- Priority Java Queue
- Queue Functions in Java
- Queue Using Array in Java
- Classes that Implement Queue
- Wrapping Up
Want to learn pro-level Java programming?
Here is our Java Course Video
{
“@context”: “https://schema.org”,
“@type”: “VideoObject”,
“name”: “Java Tutorial for Beginners”,
“description”: “Queue in Java: An Introduction with Example”,
“thumbnailUrl”: “https://img.youtube.com/vi/9HlQL8Bi1Dk/hqdefault.jpg”,
“uploadDate”: “2023-02-24T08:00:00+08:00”,
“publisher”: {
“@type”: “Organization”,
“name”: “Intellipaat Software Solutions Pvt Ltd”,
“logo”: {
“@type”: “ImageObject”,
“url”: “https://intellipaat.com/blog/wp-content/themes/intellipaat-blog-new/images/logo.png”,
“width”: 124,
“height”: 43
}
},
“contentUrl”: “https://www.youtube.com/watch?v=9HlQL8Bi1Dk”,
“embedUrl”: “https://www.youtube.com/embed/9HlQL8Bi1Dk”
}
Java Queue
A queue is a type of data structure that operates according to the ‘First-In-First-Out’ (FIFO) principle, which states that the first item entered into the queue will also be the first one taken out. This is comparable to a line in real life, where those in front of the line are the ones who will be served next.
Consider the situation where you are in charge of a bank’s customer line that is waiting to be served. As soon as they enter the bank, the clients join the back of the line. Each client leaves the line after being served. In this case, the line of customers waiting to be served is represented by a queue, with the person in front of the line being the next to be served.
The Java Collections Foundation’s Queue interface in Java offers a framework for constructing queue data structures. LinkedList, ArrayDeque, and PriorityQueue are just a few of the implementations of the Queue interface, which is an extension of the Collection interface.
Using a LinkedList as the underlying data structure, the following is an example of how you may implement this scenario:

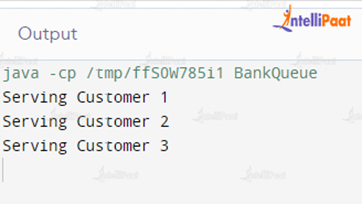
This code utilizes a LinkedList as the underlying data structure and produces a Queue named bank queue. Customers are added to the end of the line using the add method, and customers are removed from the line using the remove method so that the person in front of them may be served. Before servicing each client, the isEmpty method is used to determine if the queue is empty.
Here is our Java programming Tutorial for you.
Priority Java Queue
In a priority queue, each element has a priority and is handled in accordance with that priority. The Java class java.util.PriorityQueue can be used to implement priority queues in java.
This class implements the Queue interface and gives users a mechanism to store queue items in a prioritized order. The element with the greatest priority is the first to be dequeued, and this process continues until all elements have been dequeued.
Here is an illustration of how to utilize a Java PriorityQueue:


Learn more through our Java Certification Training Course.
Queue Functions in Java
An abstract data type called a queue depicts a collection of items in which new components are added to the back and old ones are removed from the front. The java.util package can be used to implement queues in Java. Queue interface, which specifies the number of frequently used operations that may be carried out on a queue. The following are a few of the most crucial duties:
- offer(E, e): Moves an element to the end of the queue. if the element was successfully added, returns true; otherwise, it returns false if the queue is full.
- poll(): Removes and brings back the element that is currently at the front of the queue. It returns null if the queue has no items in it.
- peek(): Without deleting it, returns the element at the front of the queue. It returns null if the queue has no items in it.
- add(E e): Adds an element to the end of the queue. Throws an IllegalStateException if the queue is full.
- remove(): Removes and returns the element at the front of the queue. Throws a NoSuchElementException if the queue is empty.
- element(): Returns the element at the front of the queue without removing it. Throws a NoSuchElementException if the queue is empty.
- isEmpty(): Returns true if the queue is empty, and false otherwise.
- size(): Returns the number of elements in the queue.
Queue Using Array in Java
A queue can be implemented using an array in Java by defining a class with the following attributes and methods:
- An integer array queue to store the elements of the queue.
- Two integer variables, front, and rear, to keep track of the front and rear of the queue.
- An integer variable size to keep track of the number of elements in the queue.
- An integer variable capacity to store the maximum number of elements that the queue can hold.
Classes that Implement Queue
There are multiple Java classes that implement the Queue interface and offer various queue types depending on how they do so:
Class LinkedList
By utilizing its offer function to enqueue entries and its poll method to dequeue elements, the LinkedList class may be utilized as a queue. A doubly-linked list may be added to and removed from at the end of the list in constant time thanks to the LinkedList class.
ArrayDeque class
The ArrayDeque class provides a dynamic array as a double-ended queue. At both ends of the queue, it offers constant-time insertions and deletions. The offerFirst and offerLast methods of the ArrayDeque class are used to enqueue elements at the front and back, respectively, while the pollFirst and pollLast methods are used to dequeue elements from the front and back, respectively.
PriorityQueue class
The PriorityQueue class implements a priority queue, where components are sorted either according to their natural ordering or by using a predetermined comparator. Because the PriorityQueue class is built as a binary heap, enqueue and dequeue operations may be completed in logarithmic time.
Preparing for interviews? You can surely refer to our Top 100+ Java Interview Questions and Answers.
Career Transition
Wrapping Up
To summarize, in a queue, items are added to the back of the line and removed from the front, adhering to the First-In-First-Out (FIFO) principle. There are several uses for queues, including resource management, communication, and scheduling tasks. A fundamental data structure in computer science, queues can be implemented in Java using a variety of methods, including built-in classes and custom arrays. Learning how queues operate and how to construct them is a crucial step in developing your Java programming skills.
The post Queue in Java: An Introduction with Example appeared first on Intellipaat Blog.
Blog: Intellipaat - Blog
Leave a Comment
You must be logged in to post a comment.