How to Use For Loop in React? (With Examples)
Before diving into the exciting world of using for loops in React, it’s important to have a solid foundation in React development. Familiarity with JavaScript, including understanding concepts like arrays and functions, is crucial.
Additionally, a working knowledge of JSX syntax and React components is essential. If you’re new to React, consider exploring introductory tutorials and gaining hands-on experience with React basics. Armed with these prerequisites, you’ll be well-prepared to unlock the true potential of for loops in your React applications.
- Introduction to Looping in Programming
- Types of Loops in JavaScript
- Working of For Loop in React
- Types of For Loops in React
- Benefits of For Loop in React
- Limitations of For Loop in React
- Conclusion
Check out our Full Stack Web Development Course on YouTube:
{
“@context”: “https://schema.org”,
“@type”: “VideoObject”,
“name”: “Full Stack Web Development Course | Full Stack Developer Course | Intellipaat”,
“description”: “How to Use For Loop in React? (With Examples)”,
“thumbnailUrl”: “https://img.youtube.com/vi/ofekyjYd0xM/hqdefault.jpg”,
“uploadDate”: “2023-07-12T08:00:00+08:00”,
“publisher”: {
“@type”: “Organization”,
“name”: “Intellipaat Software Solutions Pvt Ltd”,
“logo”: {
“@type”: “ImageObject”,
“url”: “https://intellipaat.com/blog/wp-content/themes/intellipaat-blog-new/images/logo.png”,
“width”: 124,
“height”: 43
}
},
“embedUrl”: “https://www.youtube.com/embed/ofekyjYd0xM”
}
Introduction to Looping in Programming
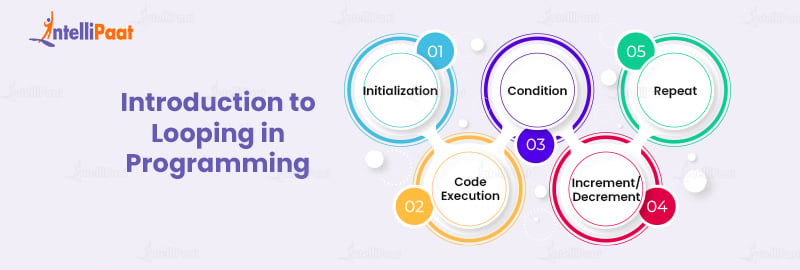
In the vast programming landscape, loops and iteration statements are essential tools that enable developers to perform repetitive tasks efficiently. When faced with scenarios where the same instructions must be executed multiple times, loops come to the rescue.
The concept behind loops revolves around the idea of iterating over a block of code until a certain condition is met. This iterative process allows for efficient execution and manipulation of data, saving developers from the burden of manually repeating the same set of instructions.
There are various types of loops available in most programming languages, including for loops, while loops, and do-while loops. Each loop type serves a specific purpose, catering to different scenarios and conditions.
The syntax of loops typically follows a similar structure across programming languages.
Below is the syntax of “for
” loop:
for (initialization; condition; increment/decrement) { // code to be executed }
The algorithm of a loop can be summarized as follows:
- Initialization: Define and initialize a control variable to a starting value.
- Condition: Evaluate a condition that determines whether the loop should continue executing or terminate.
- Code Execution: Execute the block of code within the loop.
- Increment/Decrement: Modify the control variable to progress toward the termination condition.
- Repeat: If the termination condition is still satisfied, repeat steps 3 and 4.
Loops empower programmers to handle tasks such as iterating through arrays, performing calculations on data sets, or even controlling the flow of a program based on specific conditions. By leveraging loops, developers can write concise and efficient code, enhancing productivity and reducing redundancy.
Whether it’s iterating through data structures or automating repetitive tasks, mastering the art of loops opens doors to endless possibilities and unleashes the true potential of software development.
Types of Loops in JavaScript
Let us explore the various types of loops in javascript that are as follows:
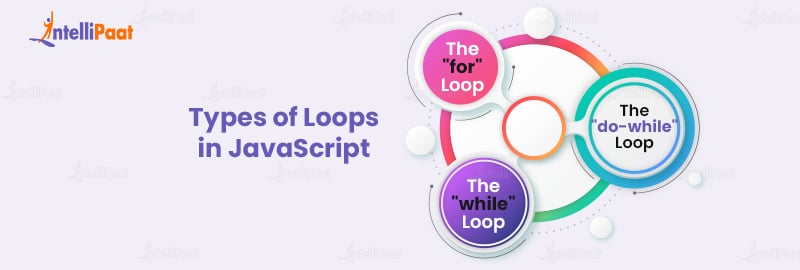
The “for” Loop
The “for
” loop is widely used and allows you to repeat a code block for a specific number of times. Its syntax consists of an initialization statement, a condition, and an increment statement.
Here’s an example:
for (let i = 0; i < 5; i++) {
// Code to be repeated
}
Algorithm:
- Initialize a counter variable (e.g., i) to the starting value.
- Set the condition for the loop to continue executing.
- Execute the code block.
- Update the counter variable.
- Repeat steps 2-4 until the condition becomes false.
The “while” Loop
The while loop repeatedly executes a block of code until a specified condition is true. Its syntax involves a condition that is evaluated before each iteration. Here’s an example:
let i = 0;
while (i < 5) {
// Code to be repeated
i++;
}
Algorithm:
- Initialize a counter variable (e.g., i) to the starting value.
- Check the condition.
- Execute a block of code until the if condition is true.
- Update the counter variable.
- Until the condition becomes false, repeat steps 2-4.
The “do-while” Loop
The working of the do-while loop is similar to the while loop, the do-while loop executes a code block as long as the specified condition is true. However, the condition is evaluated after executing the code block, ensuring that the code executes at least once.
Here’s an example:
let i = 0;
do {
// Code to be repeated
i++;
} while (i < 5);
Algorithm:
- Initialize a counter variable (e.g., i) to the starting value.
- Execute the code block.
- Update the counter variable.
- Check the condition.
- Repeat steps 2-4 until the condition becomes false.
Coding Examples
Let’s see practical examples of loops in action:
Example 1:
Printing numbers from 1 to 5 using a “for
” loop.
for (let i = 1; i <= 5; i++) {
console.log(i);
}
Output:
1
2
3
4
5
Example 2:
Summing numbers from 1 to 10 using a while loop.
let i = 1;
let sum = 0;
while (i <= 10) {
sum += i;
i++;
}
console.log(sum);
Output:
55
Example 3:
Finding the factorial of a number using a do-while loop.
let num = 5;
let factorial = 1;
let i = 1;
do {
factorial *= i;
i++;
} while (i <= num);
console.log(factorial);
Output:
120
Become an expert in React by enrolling to our React JS Course.
Working of For Loop in React
Let’s start with a beginner-level example where we will use a “for” loop to render a list of elements in React.
import React from 'react'; function ListExample() { const fruits = ['Apple', 'Banana', 'Orange', 'Mango']; const renderList = () => { const listItems = []; for (let i = 0; i < fruits.length; i++) { listItems.push({fruits[i]} ); } return listItems; }; return{renderList()}
; } export default ListExample;
In the provided code illustration, we initiate the process by establishing an array containing various fruits, with the intention of presenting them individually as separate items within a list. Our initial step entails creating an empty array named “listItems
“. Subsequently, through the utilization of a “for
” loop, we iterate over each fruit within the array, generating an
listItems
” array. Finally, we present the resulting array of list items encapsulated within an
- element.
Now, let us delve into a more sophisticated scenario that involves employing a “for
” loop to filter data based on a specific condition.
import React from 'react';
function FilterExample() {
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const filteredNumbers = [];
const filterData = () => {
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
filteredNumbers.push(numbers[i]);
}
}
};
filterData();
return{filteredNumbers.join(', ')};
}
export default FilterExample;
In the above coding example, we have used an array of numbers, and the aim is to filter out the even numbers. We initialize an empty array called filteredNumbers. Using a “for
” loop, we iterate over the numbers array, and if a number is divisible by 2 (i.e., it’s even), we push it into the filteredNumbers array. Finally, we render the filtered numbers joined by commas.
Types of For Loops in React
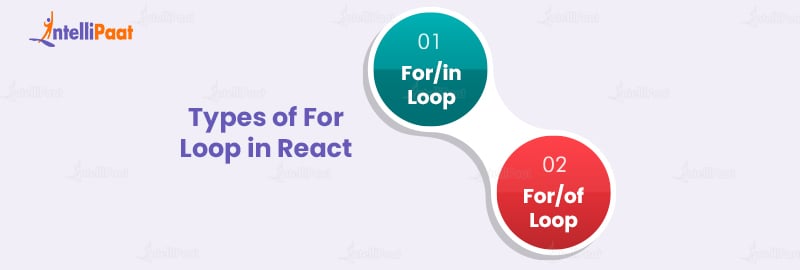
For/in Loop
In React, the “for/in
” loop is a handy construct that allows you to iterate over the properties of an object. Also, on the elements of an array. This loop provides an efficient way to access and manipulate data in React components.
Syntax of the "for/in
" Loop:
The syntax of the “for/in
” loop in React is as follows:
for (variable in object) {
// Code to be executed for each property/element
}
The “variable
” represents a different property or element on each iteration, while the “object
” is the object or array you want to loop through.
Code Example and Explanation:
Let’s turn our gaze to a code example to understand how the “for/in
” loop works in React:
import React from 'react'; function LoopExample() { const user = { name: 'John Doe', age: 25, email: 'johndoe@example.com', occupation: 'Developer', }; const renderUserDetails = () => { const userDetails = []; for (const key in user) { userDetails.push({key}: {user[key]}
); } return userDetails; }; return{renderUserDetails()}; } export default LoopExample;
In the provided illustration, an object named “user
” is presented, encompassing properties such as name, age, email, and occupation. To depict this, a function named “renderUserDetails
” is established, initializing an empty array named “userDetails
“. Employing the “for/in
” loop, we systematically iterate through each property of the “user
” object. For every property encountered, a paragraph element is generated, comprising the property name as a label and its associated value. In order to maintain uniqueness, a distinctive key is assigned to each paragraph element using the property name. Ultimately, the “userDetails
” array is returned.
Applications of the “for/in” Loop in React:
- Rendering Dynamic Lists: The “for/in” loop can be used to dynamically render lists of data fetched from an API or stored in an object or array.
- Form Generation: If you have an object representing form fields, you can use the “for/in” loop to dynamically generate form elements based on the object’s properties.
- Data Manipulation: The “for/in” loop is helpful for iterating through an object or array and performing various data manipulation tasks, such as filtering, sorting, or transforming the data.
- Dynamic Styling: You can use the “for/in” loop to apply dynamic styling to elements based on their properties.
- Object Property Validation: The “for/in” loop allows you to check the existence or validity of specific properties within an object and perform appropriate actions based on the validation results.
For/of Loop
The “for/of” loop in React is a powerful construct that allows you to iterate over iterable objects, such as arrays or strings. It provides a concise and elegant way to access and process each element of the iterable.
Syntax of the “for/of” Loop:
The syntax of the “for/of” loop in React is as follows:
for (variable of iterable) {
// Code to be executed for each element
}
The “variable
” represents a different element on each iteration, while the “iterable” is the array or iterable object you want to loop through.
Code Example and Explanation:
Here, look at the coding example to understand how the “for/of” loop works in React:
import React from 'react';
function LoopExample() {
const fruits = ['Apple', 'Banana', 'Orange', 'Mango'];
const renderFruits = () => {
const fruitList = [];
for (const fruit of fruits) {
fruitList.push({fruit} );
}
return{fruitList}
;
};
return{renderFruits()};
}
export default LoopExample;
We have an array called “fruits
” that contains different fruit names. We define a function called “renderFruits
” that initializes an empty array called “fruitList
“. Using the “for/of
” loop, we iterate through each element of the “fruits” array. For each fruit, we create a list item element with the fruit name as the content. We assign a unique key to each list item element using the fruit name. Finally, we return the “fruitList
” array wrapped in a
- element.
- String Manipulation: If you have a string, the “
for/of
” loop allows you to access and process each character individually, enabling string manipulation tasks like searching, replacing, or formatting. - Iterating over React Children: In React, it can be used to iterate over the children of a component and apply operations to each child individually.
- Working with Iterable Objects: The “for/of” loop is not limited to arrays; it can also be used with other iterable objects, such as sets or maps, to iterate over their elements.
- Dynamic Rendering of Components: One of the primary advantages of loops in React is their ability to render components based on data dynamically. By iterating through an array or object, you can generate components on the fly, saving you from writing repetitive code. This feature is useful when dealing with dynamic data or rendering large lists.
- Efficient Data Manipulation: Loops in React enable developers to manipulate data with ease. Whether it’s filtering, mapping, or transforming data, loops provide a concise and efficient way to perform these operations. By iterating through the data, you can apply logic and modify it according to your application’s requirements.
- Code Reusability: Loops promote code reusability in React. Instead of writing similar code multiple times, you can encapsulate the logic within a loop and reuse it whenever needed. This not only reduces redundancy but also makes the code more maintainable.
- Scalability: React applications become more scalable with loops. As your data increases, loops facilitate in handling larger datasets without worrying about performance issues. These loops efficiently iterate through the data and help keep the application responsive and agile, regardless of the size of the data. With these loops in place, your React applications will be well-equipped to handle any growing data and be able to quickly adjust to it, so that performance is never compromised.
- Flexibility in Data Structures: Loops provide flexibility when working with different data structures in React. Whether it’s an array, object, or even nested data, loops allow you to traverse and process the data structure effortlessly. This flexibility opens up possibilities for complex data manipulation and access to nested properties.
- Conditional Rendering: Loops combined with conditional statements offer powerful options for conditional rendering in React. By incorporating logic within the loop, you can conditionally render components or apply different styles based on specific criteria. This dynamic rendering capability enhances the user experience and improves the overall interactivity of your application.
- Optimization and Performance: Loops in React play a vital role in optimizing code and improving performance. By using loops, you can minimize redundant code and ensure that operations are executed only when necessary. This optimization reduces the computational load on the application, resulting in faster rendering and improved efficiency.
- Lack of Declarative Approach: React encourages a declarative approach to building user interfaces. The “
for
” loop, being an imperative construct, can hinder the ability to express UI components declaratively. In React, it is often more advantageous to utilize higher-order array methods like map, filter, or reduce that provide a more concise and declarative syntax. - Difficulty with Asynchronous Operations: The “
for
” loop is not well-suited for handling asynchronous operations, such as making API requests or fetching data from a server. As the loop executes synchronously, it may cause blocking behaviour and impact the responsiveness of your application. In such cases, it’s better to use asynchronous patterns like Promise.all, async/await, or fetch to manage asynchronous operations in a more controlled manner. - Limited Support for React Components: The “
for
” loop is primarily designed for iterating over arrays or objects and performing operations at a lower level. When it comes to rendering React components dynamically, using a “for
” loop might lead to difficulties in managing component state, handling key assignments, or leveraging the component lifecycle effectively. React provides specialized tools like the map function or JSX syntax to handle component rendering more efficiently. - Performance Implications: In large-scale applications with extensive data manipulation requirements, the “
for
” loop might not be the most performant option. Iterating over large arrays using a “for
” loop can result in slower execution times compared to optimized alternatives like map or filter. These higher-order array methods leverage internal optimizations and can yield better performance in most cases. - Lack of Built-in Immutability: React strongly encourages immutability to facilitate efficient rendering and state management. The “
for
” loop does not inherently provide immutability guarantees, and modifying objects or arrays within a loop can lead to unintended side effects and make it difficult to track changes. Immutability libraries like Immutable.js or immutability patterns like spread operators (…) can be used to ensure data integrity and optimize React’s rendering process. - Incompatibility with JSX: JSX, the JavaScript syntax extension used in React, introduces a syntax mismatch when directly incorporating a “
for
” loop within JSX code. Mixing the “for
” loop syntax with JSX syntax can result in confusing code structure and make the code harder to read and maintain. Utilizing higher-order array methods like a map or filter with JSX expressions can provide cleaner and more readable code. - Limited Error Handling: The “
for
” loop does not provide built-in error handling mechanisms. If an error occurs within the loop, it can potentially break the loop and halt the execution of subsequent iterations. In contrast, higher-order array methods often come with error-handling mechanisms, such as try-catch blocks, that allow for more robust error management.
Applications of the “for/of
” Loop in React:
Career Transition
Benefits of For Loop in React
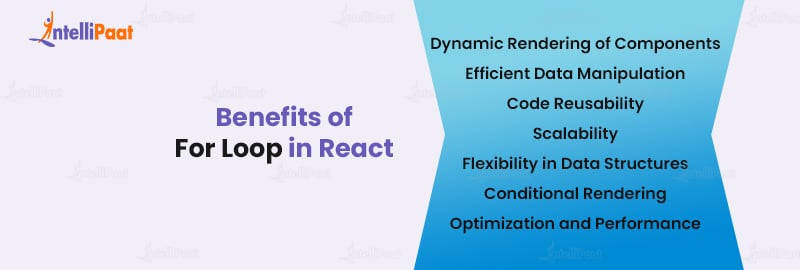
Let’s explore the key benefits of utilizing loops in React that will provide deep understanding about the iteration process in React:
Get ready for your next React interview by going through the most asked React Interview Questions.
Limitations of For Loop in React
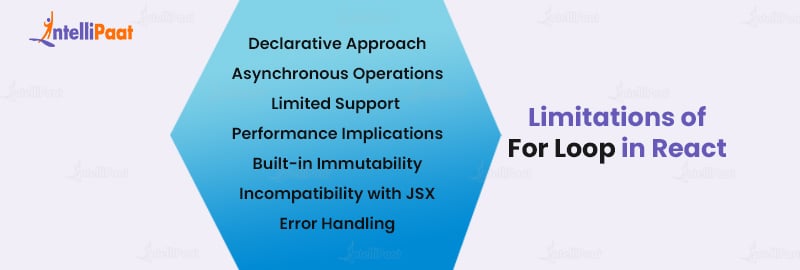
Below listed are some of the drawbacks associated with the usage of the “for
” loop in React:
Conclusion
Understanding how to use a “for” loop in React is an essential skill for any developer working with this JavaScript library. By utilizing a “for” loop, we can efficiently iterate over arrays or perform repetitive tasks in our React applications. Throughout this article, we have explored various examples that demonstrate the practical implementation of “for” loops in React components. From rendering dynamic lists to fetching data from APIs, “for” loops provide us with a powerful tool for managing and manipulating data. With this newfound knowledge, you can enhance the functionality and interactivity of your React applications, making them more robust and user-friendly.
Have any query for React to get cleared? Then go through our Web Community.
The post How to Use For Loop in React? (With Examples) appeared first on Intellipaat Blog.
Blog: Intellipaat - Blog
Leave a Comment
You must be logged in to post a comment.