How to Build a React Native Image Picker?
Before starting on your journey of building a React Native Image Picker, it is essential to ensure you have a solid foundation. Prerequisites include a basic understanding of React Native development, familiarity with JavaScript and React concepts, and a working development environment with installed Node.js and a code editor. Also, knowledge of handling permissions, accessing the device’s photo library, and installing necessary dependencies like react-native-image-picker will be advantageous.
Table of Contents:
- What is React Native Image Picker?
- Benefits of Building a React Native Image Picker
- Building a React Native Image Picker
- What are the Applications of Image Picker?
- Conclusion
Watch this ReactJS full course video to learn more about ReactJS:
{
“@context”: “https://schema.org”,
“@type”: “VideoObject”,
“name”: “ReactJS Full Course | ReactJS Tutorial for Beginners | ReactJS Training | Intellipaat”,
“description”: “How to Build a React Native Image Picker?”,
“thumbnailUrl”: “https://img.youtube.com/vi/MwgWuzO7fHs/hqdefault.jpg”,
“uploadDate”: “2023-07-21T08:00:00+08:00”,
“publisher”: {
“@type”: “Organization”,
“name”: “Intellipaat Software Solutions Pvt Ltd”,
“logo”: {
“@type”: “ImageObject”,
“url”: “https://intellipaat.com/blog/wp-content/themes/intellipaat-blog-new/images/logo.png”,
“width”: 124,
“height”: 43
}
},
“embedUrl”: “https://www.youtube.com/embed/MwgWuzO7fHs”
}
What is React Native Image Picker?
React Native Image Picker is a robust tool that empowers developers to incorporate image selection functionality seamlessly into their React Native applications. It allows users to select images from their device’s photo library or capture new ones via the camera.
- Using React Native Image Picker, developers can elevate their applications by allowing users to upload, manipulate, and exhibit images within the app’s interface.
- This tool is a valuable asset, enhancing user engagement and expanding the range of visual content that can be utilized within the application
- React Native Image Picker bridges the native code of iOS and Android platforms with the JavaScript code of React Native.
- It uses platform-specific APIs and libraries to access the device’s photo library and camera, allowing users to select images familiarly and intuitively.
- React Native Image Picker tool allows developers to build cross-platform applications with consistent image selection capabilities across iOS and Android devices.
- The React Native Image Picker library provides easy-to-use methods and components that simplify the process of integrating image selection functionality.
- Developers can access the device’s photo library, capture images using the camera, or even select multiple images simultaneously.
- It offers various configuration options, allowing developers to specify the allowed media types, set image quality, define cropping parameters, and more.
- React Native Image Picker also provides callbacks and events that allow developers to handle the selected images and perform actions such as uploading, editing, or displaying them within the apps.
Enroll now in the React JS course to learn its concepts from experts.
Benefits of Building a React Native Image Picker
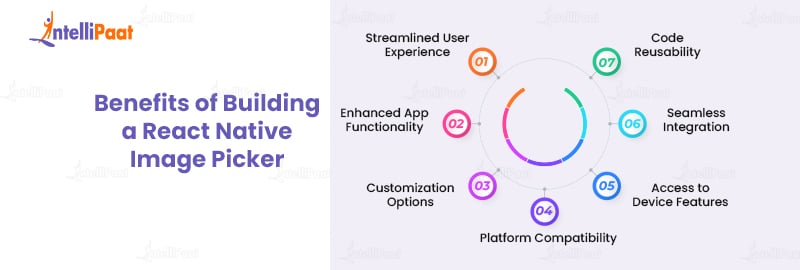
Let’s explore the top benefits of building a React Native Image Picker:
- Streamlined User Experience: By incorporating an Image Picker, you empower users to seamlessly select images without leaving the app. This streamlined experience eliminates the need for users to switch between apps or access their photo gallery separately, resulting in a smoother and more efficient workflow.
- Enhanced App Functionality: With an Image Picker, you can enrich your application’s functionality by allowing users to upload images as part of their interactions. This opens up possibilities for social media apps, e-commerce platforms, and content creation tools, enabling users to easily share and engage with visual content.
- Customization Options: Building your own Image Picker gives you full control over its design and behavior. You can customize the user interface, add animations, and tailor the user experience to align with your app’s branding and visual aesthetics. This level of customization ensures a cohesive and consistent experience for your users.
- Platform Compatibility: React Native Image Pickers are platform-agnostic, meaning they work seamlessly across both iOS and Android devices. By utilizing a single codebase, you save development time and effort while still delivering a consistent experience to users across different platforms.
- Access to Device Features: An Image Picker grants access to various device features like a camera, photo library, and gallery.
- Seamless Integration with Other Libraries: The React Native ecosystem offers a vast array of third-party libraries that can be seamlessly integrated with an Image Picker. These libraries provide advanced functionalities such as image editing, image cropping, image compression, and more. Leveraging these libraries enhances the versatility and richness of your Image Picker, making it even more powerful.
- Code Reusability: One of the key benefits of using React Native is code reusability. You can easily integrate an Image Picker into multiple projects by building an Image Picker as a reusable component. This saves development time and ensures consistency and efficiency across your applications.
Check out our blog on ReactJS Tutorial to learn more about ReactJS.
Building a React Native Image Picker
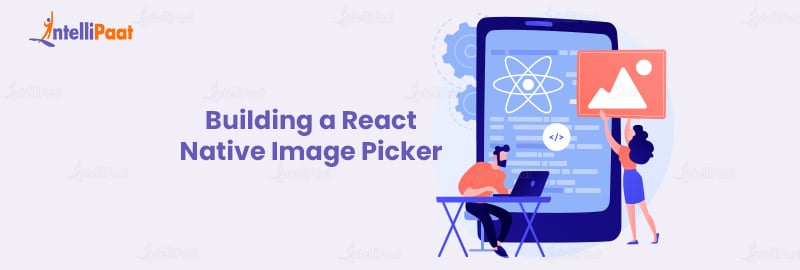
Step 1: Choosing React Native Image Picker
There are two well-known libraries available for implementing the image picker component in React Native:
react-native-image-picker
react-native-image-crop-picker
Which one to choose?
While React Native Image Picker is a popular module for selecting media from the device library or camera, React Native Image Crop Picker offers an additional advantage.
- The distinct advantage of using react-native-image-crop-picker is its ability to crop and compress images.
- This feature becomes particularly significant when developing iOS apps, as uploading large files can potentially impact performance.
- By compressing and cropping images, developers can mitigate such issues and improve the overall user experience, especially for users with slower internet speeds.
Step 2: Create a new React Native project using typescript.
npx react-native init ImagePickerDemo --template react-native-template-typescript
Or,
import ImagePicker from 'react-native-image-crop-picker';
Step 3: Add the build.gradle android configuration.
allprojects { repositories { mavenLocal() center() maven { url "$rootDir/../node_modules/react-native/android" } // ADD THIS maven { url 'https://maven.google.com' } // ADD THIS maven { url "https://www.jitpack.io" } } } Add useSupportLibrary (android/app/build.gradle) android { ... defaultConfig { ... vectorDrawables.useSupportLibrary = true ... } ... } Use Android SDK >= 26 (android/app/build.gradle) android { compileSdkVersion 27 buildToolsVersion "27.0.3" ... defaultConfig { ... targetSdkVersion 27 ... } ... }
Step 4: Building the profile avatar
import React from 'react'; import {Image, ImageProps, StyleSheet, TouchableOpacity} from 'react-native'; import ImagePicker, {ImageOrVideo} from 'react-native-image-crop-picker'; interface AvatarProps extends ImageProps { onChange?: (image: ImageOrVideo) => void; } export const Avatar = (props: AvatarProps) => { const [uri, setUri] = React.useState(props.source?.uri || undefined); const pickPicture = () => { ImagePicker.openPicker({ width: 300, height: 400, cropping: true, }).then(image => { setUri(image.path); props.onChange?.(image); }); }; return ( ); }; const styles = StyleSheet.create({ avatar: { paddingTop: 20, height: 100, width: 100, borderRadius: 100, padding: 20, }, });
Step 5: Select pictures and videos from the device’s gallery or camera.
To use the camera, we only need to call the openCamera function instead of openPicker:
import React from 'react'; import { Image, ImageProps, Pressable, SafeAreaView, StyleSheet, Text, TouchableOpacity, } from 'react-native'; import ImagePicker, {ImageOrVideo} from 'react-native-image-crop-picker'; import Modal from 'react-native-modal'; import {CameraIcon, ImageIcon} from './icons'; interface AvatarProps extends ImageProps { onChange?: (file: ImageOrVideo) => void; } export const Avatar = (props: AvatarProps) => { const [uri, setUri] = React.useState(props.source?.uri || undefined); const [visible, setVisible] = React.useState(false); const close = () => setVisible(false); const open = () => setVisible(true); const chooseImage = () => { ImagePicker.openPicker({ width: 300, height: 400, cropping: true, }) .then(image => { setUri(image.path); props.onChange?.(image); }) .finally(close); }; const openCamera = () => { ImagePicker.openCamera({ width: 300, height: 400, cropping: true, }) .then(image => { setUri(image.path); props.onChange?.(image); }) .finally(close); }; return ( Library Camera ); ; const styles = StyleSheet.create({ avatar: { paddingTop: 20, height: 100, width: 100, borderRadius: 100, padding: 20, }, options: { backgroundColor: 'white', flexDirection: 'row', borderTopRightRadius: 30, borderTopLeftRadius: 30, }, option: { flex: 1, justifyContent: 'center', alignItems: 'center', }, });
- Single image selection from the device’s gallery
ImagePicker.openPicker({ width: 300, height: 400, cropping: true }).then(image => { console.log(image); });
- Multiple image selections from the device’s gallery
ImagePicker.openPicker({ multiple: true }).then(images => { console.log(images); });
- Video selection from the device’s gallery
ImagePicker.openPicker({ mediaType: "video", }).then((video) => { console.log(video); });
- Single image selection from the device’s camera
ImagePicker.openCamera({ width: 300, height: 400, cropping: true, }).then(image => { console.log(image); });
- Video selection from the device’s camera
ImagePicker.openCamera({ mediaType: 'video', }).then(image => { console.log(image); });
Step 6: Final code
import * as React from 'react'; import {View} from 'react-native'; import {ImageOrVideo} from 'react-native-image-crop-picker'; import {Avatar} from './Avatar'; export const Profile = () => { const onAvatarChange = (image: ImageOrVideo) => { console.log(image); // upload image to server here }; return ( ); };
Career Transition
What are the Applications of Image Picker?
Let’s explore the top applications of Image Picker and how it enhances user experiences in different contexts.
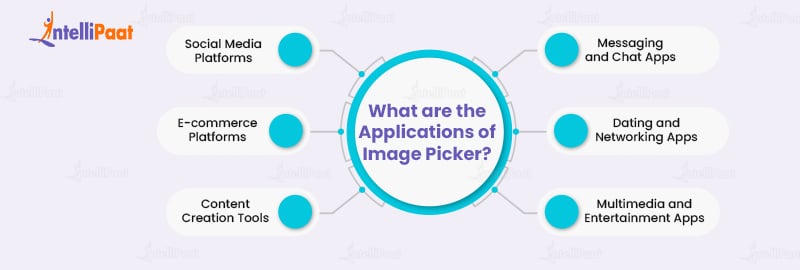
- Social Media Platforms: Social media apps heavily rely on visual content. Users can easily select and upload images to their profiles, posts, or stories with an Image Picker. This feature enables users to effortlessly share their moments, experiences, and creativity, fostering engagement and interaction within the social media community.
- E-commerce Platforms: Image selection is crucial in e-commerce applications. Users can upload product images by integrating an Image Picker, allowing sellers to showcase their products effectively. Customers can also share images of purchased products, enhancing the overall shopping experience by providing visual feedback to other users.
- Content Creation Tools: Image-based content creation tools, such as graphic design or photo editing apps, heavily rely on Image Pickers. Users can select images from their device’s library to create collages, edit photos, or design visually appealing graphics. The Image Picker feature offers seamless integration, allowing users to access their images within the app and unleash their creativity.
- Messaging and Chat Apps: Image Pickers play a vital role in messaging and chat apps. Users can share images instantly from their photo gallery or capture images using the camera. This feature enables users to express themselves, share moments, or convey information visually, enriching the communication experience and making it more engaging and dynamic.
- Dating and Networking Apps: In dating and networking apps, profile pictures are crucial. With an Image Picker, users can easily select and upload their desired profile pictures, enhancing their online presence. Choosing images directly within the app saves time and effort, enabling users to create a captivating profile and make a memorable first impression.
- Multimedia and Entertainment Apps: Multimedia and entertainment apps, such as video editing or streaming platforms, often require the selection of images for thumbnails, cover art, or video overlays. An Image Picker streamlines this process, allowing users to conveniently choose images and enhance the visual presentation of their multimedia content.
Preparing for interviews? Check out this React Interview Questions list!
Conclusion
Building a React Native Image Picker can greatly enhance the functionality and user experience of your applications. By following the step-by-step process outlined in this tutorial, you can seamlessly integrate an image selection feature into your React Native projects. From handling permissions to accessing the device’s photo library and utilizing third-party libraries like react-native-image-picker, you now possess the knowledge and tools to create a robust and user-friendly image picker.
Embrace the power of visual media in your apps and provide your users with a convenient and delightful image selection experience. Start building your own React Native Image Picker today and unlock new possibilities for your applications.
Still in doubt? Put your query on Intellipaat’s community page.
The post How to Build a React Native Image Picker? appeared first on Intellipaat Blog.
Blog: Intellipaat - Blog
Leave a Comment
You must be logged in to post a comment.