Bitwise Operators in C Programming
In this comprehensive guide, we will explore the world of bitwise operators in C, exploring their significance, types, use cases, and practical examples.
Here are the following topics we are going to cover:
- What is a Bitwise Operator in C Programming?
- Types of Bitwise Operators in C Programming
- Examples of Bitwise Operators in C
- Use Cases of Bitwise Operators
- Conclusion
Learn data structures and algorithms in this comprehensive video course:
{
“@context”: “https://schema.org”,
“@type”: “VideoObject”,
“name”: “Data Structures And Algorithms | DSA Course | Data Structures And Algorithms Tutorial | Intellipaat”,
“description”: “Bitwise Operators in C Programming”,
“thumbnailUrl”: “https://img.youtube.com/vi/3Aid2c1QafI/hqdefault.jpg”,
“uploadDate”: “2023-09-05T08:00:00+08:00”,
“publisher”: {
“@type”: “Organization”,
“name”: “Intellipaat Software Solutions Pvt Ltd”,
“logo”: {
“@type”: “ImageObject”,
“url”: “https://intellipaat.com/blog/wp-content/themes/intellipaat-blog-new/images/logo.png”,
“width”: 124,
“height”: 43
}
},
“embedUrl”: “https://www.youtube.com/embed/3Aid2c1QafI”
}
What is a Bitwise Operator in C Programming?
Bitwise operators are fundamental operators in the C programming language that allow you to perform operations on individual bits of integer-type variables. These operators work at a low level, directly manipulating the binary representation of data. Bitwise operators are particularly useful for tasks that involve bit manipulation, setting or clearing specific bits, creating bit masks, and optimizing memory usage.
These operators find their primary application with integer types like int, unsigned int, and char, proving exceptionally advantageous in contexts such as embedded systems, low-level programming, hardware communication, and specific optimization scenarios. There are mainly six types of bitwise operators in C programming that include AND (&), OR (|), XOR (^), NOT (~), left shift (<>).
Truth Table of Bitwise Operators
Let us now understand the truth table that shows the results of common bitwise operators for two binary inputs, A and B:
A | B | A & B | A | B | A ^ B | ~A | ~B |
0 | 0 | 0 | 0 | 0 | 1 | 1 |
0 | 1 | 0 | 1 | 1 | 1 | 0 |
1 | 0 | 0 | 1 | 1 | 0 | 1 |
1 | 1 | 1 | 1 | 0 | 0 | 0 |
In the table,
- A & B represents the bitwise AND operation between A and B.
- A | B represents the bitwise OR operation between A and B.
- A ^ B represents the bitwise XOR operation between A and B.
- ~A represents the bitwise NOT operation on A.
- ~B represents the bitwise NOT operation on B.
In each cell of the table, the values represent the result of the corresponding operation when applied to the given inputs.
Are you interested in becoming a full-stack developer? Take our Full Stack Development Course to take your first step.
Types of Bitwise Operators in C Programming
In the domain of C programming, various types of bitwise operators are available for manipulating the individual bits of integers. Let us now explore each type in detail:
Bitwise AND (&)
The bitwise AND (&) operator in C performs an operation between the individual bits of two integers. It results in a new integer where each bit position is set to 1 only if both corresponding bits in the input integers are 1. If any of the corresponding bits are 0, the resulting bit will be set to 0.
Code Example:
#include
int main() {
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int result = a & b;
printf("Result of Bitwise AND Operator is: %dn", result);
return 0;
}
Output:
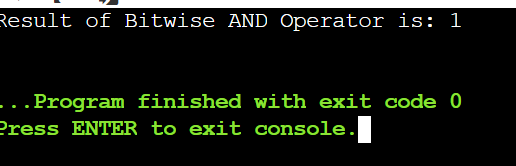
Explanation:
The provided C code snippet demonstrates the application of the bitwise AND operator on two integer variables a and b. This operator operates on the individual bits of these integers, producing a new integer with corresponding bits determined by the bitwise AND comparison of the bits in a and b.
Initially, a is assigned the value 5, which in binary is represented as 0101, and b is assigned the value 3, corresponding to the binary representation 0011.
When the bitwise AND operation a & b is performed, it involves comparing each pair of corresponding bits between a and b. Starting from the rightmost bit, the AND operation is applied:
- The first bit of a is 0, and the first bit of b is 0, resulting in 0.
- The second bit of a is 1, and the second bit of b is 0, resulting in 0.
- The third bit of a is 0, and the third bit of b is 1, resulting in 0.
- The fourth bit of a is 1, and the fourth bit of b is 1, resulting in 1.
Thus, the new integer result is constructed as 0001 in binary, which is equivalent to the decimal value 1. The code then employs the printf function to display the outcome of the bitwise AND operation. The formatted string “Result of Bitwise AND Operator is: %dn” includes a placeholder %d that is substituted with the value of result, which is 1 in this case.
Upon execution, the program produces the output “Result of Bitwise AND Operator is: 1”, affirming that the bitwise AND operation between a and b yields the result of 1, as deduced from the binary comparisons described earlier.
Bitwise OR (|)
The bitwise OR (|) operator in C is used to perform an operation on the individual bits of two integers. It produces a new integer where each bit position is set to 1 if at least one of the corresponding bits in the input integers is 1. If both corresponding bits are 0, the resulting bit will be 0.
Code Example:
#include
int main() {
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int result = a | b;
printf("Result of Bitwise OR Operator is: %dn", result);
return 0;
}
Output:
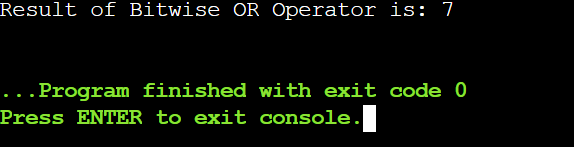
Explanation:
The provided C code snippet exemplifies the application of the bitwise OR operator to two integer variables, a and b. This operator functions by manipulating the individual bits of these integers, resulting in a new integer where each bit is determined through a bitwise OR comparison of the corresponding bits in a and b.
At the outset, a is assigned the decimal value of 5, which corresponds to the binary representation 0101. On the other hand, b is assigned the decimal value of 3, corresponding to the binary representation 0011.
Upon executing the bitwise OR operation a | b, the operation unfolds by comparing each corresponding pair of bits between a and b. Commencing from the rightmost bit, the OR operation is applied:
- The first bit of a is 0, and the first bit of b is 0, leading to a result of 0.
- The second bit of a is 1, and the second bit of b is 0, resulting in a value of 1.
- The third bit of a is 0, and the third bit of b is 1, leading to a result of 1.
- The fourth bit of a is 1, and the fourth bit of b is 1, culminating in a value of 1.
This culminates in the new integer result being constructed as 0111 in binary form, which corresponds to the decimal value 7. The code subsequently utilizes the printf function to display the outcome of the bitwise OR operation. The formatted string “Result of Bitwise OR Operator is: %dn” features a placeholder %d that gets substituted with the value of result, which in this case is 7.
Upon execution, the program generates the output “Result of Bitwise OR Operator is: 7”, confirming that the application of the bitwise OR operator to a and b yields a result of 7. This outcome aligns with the binary comparisons previously described.
Bitwise XOR (^)
The bitwise XOR (^) operator in C is utilized to perform an operation on the individual bits of two integers. It generates a new integer where each bit position is set to 1 if the corresponding bits in the input integers are different: one bit is 0 and the other bit is 1. If both corresponding bits are either 0 or 1, the resulting bit will be 0.
Code Example:
#include
int main() {
int a = 5; // Binary: 0101
int b = 3; // Binary: 0011
int result = a ^ b;
printf("Result of Bitwise XOR Operator is: %dn", result);
return 0;
}
Output:
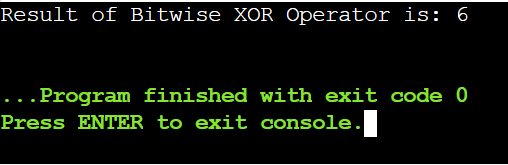
Explanation:
The provided C code demonstrates the use of the bitwise XOR operator (^) on two integer variables, a and b. The binary representations of a and b are 0101 and 0011, respectively.
When the bitwise XOR operation a ^ b is executed, it involves comparing each corresponding pair of bits between a and b:
- The first bit of a is 0, and the first bit of b is 0. The XOR operation results in 0.
- The second bit of a is 1, and the second bit of b is 0. The XOR operation yields 1.
- The third bit of a is 0, and the third bit of b is 1. The XOR operation leads to a value of 1.
- The fourth bit of a is 1, and the fourth bit of b is 1. The XOR operation gives a result of 0.
When we put these results together, we get the binary value 0110, which is equal to the decimal value 6. The code then uses the printf function to display the outcome of the bitwise XOR operation. The formatted string “Result of Bitwise XOR Operator is: %dn” contains a placeholder %d that is replaced with the value of result, which is 6 in this case.
Upon execution, the program should produce the output “Result of Bitwise XOR Operator is: 6”. This correctly indicates that the application of the bitwise XOR operator to a and b yields a result of 6.
Bitwise NOT (~)
The bitwise NOT (~) operator in C is used to perform an operation on a single integer, inverting all of its individual bits. Each bit that was originally set (1) becomes unset (0), and each bit that was originally unset (0) becomes set (1). In simple terms, the bitwise NOT operator flips the binary representation of an integer, turning all 1s into 0s and all 0s into 1s.
Code Example:
#include
int main() {
int a = 5; // Binary: 0101
int result = ~a;
printf("Result of Bitwise NOT Operator is: %dn", result);
return 0;
}
Output:
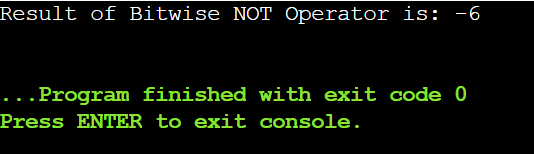
Explanation:
The provided C code exemplifies the use of the bitwise NOT (~) operator on an integer variable a. This operator operates on the individual bits of the integer, effectively inverting each bit. As a result, every bit that was originally set to 1 becomes 0, and every bit that was originally 0 becomes 1.
In this code, a is initialized with the decimal value 5, which corresponds to the binary representation 0101. When the bitwise NOT operation ~a is executed, it inverts all the bits within a:
- The first bit of a is 0, and after the NOT operation, it becomes 1.
- The second bit of a is 1, and after the NOT operation, it becomes 0.
- The third bit of a is 0, and after the NOT operation, it becomes 1.
- The fourth bit of a is 1, and after the NOT operation, it becomes 0.
This inversion process results in a new binary representation 1010. In decimal terms, this corresponds to the value -6 due to two’s complement representation.
The code utilizes the printf function to display the outcome of the bitwise NOT operation. The formatted string “Result of Bitwise NOT Operator is: %dn” includes a placeholder %d that is substituted with the value of result, which is -6 in this case.
Upon execution, the program produces the output “Result of Bitwise NOT Operator is: -6”. This affirms that applying the bitwise NOT operator to the value of a yields a result of -6, aligning with the binary inversion explained earlier.
Learn what a Linear Data Structure is and how it works!
Left Shift (<<)
The left shift (<<) operator in C is employed to shift the bits of an integer toward the left by a specified number of positions. It effectively multiplies the integer by 2, raised to the power of the shift count. The vacant positions introduced by the shift are filled with zeros.
Code Example:
#include
int main() {
int a = 5; // Binary: 0101
int result = a << 2;
printf("Result of Bitwise Left Shift Operator is: %dn", result);
return 0;
}
Output:
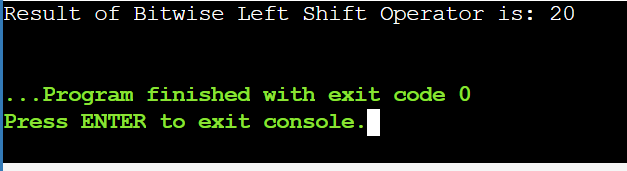
Explanation:
The provided C code demonstrates the utilization of the bitwise left shift (<<) operator on an integer variable a. This operator facilitates the shifting of individual bits within the integer toward the left by a designated number of positions. This leftward shift effectively multiplies the binary value by 2, raised to the power of the shift count.
Within the code, a is assigned the decimal value 5, which corresponds to the binary representation 0101. Upon the execution of the bitwise left shift operation a << 2, the bits within a are shifted two positions to the left:
- The first bit of a is 0, and after the left shift, it remains 0 in its new position.
- The second bit of a is 1, and after the left shift, it becomes the third bit (0100).
- The third bit of a is also 0, and after the left shift, it becomes the fourth bit (0000).
- The fourth bit of a is 1, and after the left shift, it becomes the fifth bit (1000).
This results in the new binary representation 010100, which is equivalent to the decimal value 20. This outcome is achieved by virtually multiplying the original value of a by 2, raised to the power of 2, as 2^2 is equal to 4.
The code incorporates the printf function to present the outcome of the bitwise left shift operation. The formatted string “Result of Bitwise Left Shift Operator is: %dn” includes a placeholder %d that is substituted with the value of result, which is 20 in this scenario.
Upon execution, the program should generate the output “Result of Bitwise Left Shift Operator is: 20”. This serves as confirmation that the application of the bitwise left shift operator to the value of a yields a result of 20. This aligns accurately with the binary shifting process elaborated upon earlier.
Right Shift (>>)
The right shift (>>) operator in C is used to shift the bits of an integer toward the right by a specified number of positions. It effectively divides the integer by 2, raised to the power of the shift count. The vacant positions introduced by the shift are usually filled according to the sign bit (in the case of signed integers) or with zeros (in the case of unsigned integers).
Code Example:
#include
int main() {
int a = 16; // Binary: 10000
int result = a >> 2;
printf("Result of Bitwise Right Shift Operator is: %dn", result);
return 0;
}
Output
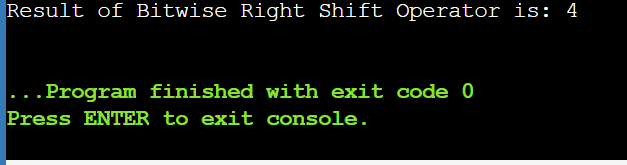
Explanation:
The provided C code demonstrates the usage of the bitwise right shift (>>) operator on an integer variable a. This operator is utilized to shift the individual bits of the integer to the right by a specified number of positions. This rightward shift is akin to dividing the binary value by 2, raised to the power of the shift count.
In the context of the code, a is initialized with the decimal value 16, which corresponds to the binary representation 10000. Upon the execution of the bitwise right shift operation a >> 2, the bits within a are shifted two positions to the right:
- The first bit of a is 1, and after the right shift, it remains 1 in its new position.
- The second bit of a is 0, and after the right shift, it becomes the first bit (01).
- The third bit of a is also 0, and after the right shift, it becomes the second bit (001).
- The fourth bit of a is 0, and after the right shift, it becomes the third bit (0001).
- The fifth bit of a is 0, and after the right shift, it becomes the fourth bit (00001).
This leads to a new binary representation, 00001, which corresponds to the decimal value 4. This outcome is achieved through a process akin to dividing the original value of a by 2, raised to the power of 2 (which equals 4).
The code employs the printf function to display the outcome of the bitwise right shift operation. The formatted string “Result of Bitwise Right Shift Operator is: %dn” incorporates a placeholder %d that is substituted with the value of result, which is equal to 4 in this case.
Upon execution, the program produces the output “Result of Bitwise Right Shift Operator is: 4”. This confirms that applying the bitwise right shift operator to the value of a yields a result of 4. This aligns accurately with the binary shifting process described earlier.
Do you want a comprehensive list of interview questions? Here are the full stack developer interview questions!
Examples of Bitwise Operators in C
Here are some common examples of how you can use bitwise operators in C:
- Setting a Bit: You can use the bitwise OR operator (|) to set a specific bit to 1 without affecting the other bits.
Syntax:
// Set the nth bit (indexing from right, starting at 0)
unsigned int mask = 1 << n;
num |= mask; // Set the nth bit
- Clearing a Bit: You can use the bitwise AND operator (&) to clear a specific bit by masking it with zeros.
Syntax:
// Clear the nth bit (indexing from right, starting at 0)
unsigned int mask = ~(1 << n); // Create a mask with nth bit as 0
num &= mask; // Clear the nth bit
- Toggling a Bit: You can use the bitwise XOR operator (^) to toggle a specific bit (change 0 to 1 or 1 to 0).
Syntax:
// Toggle the nth bit (indexing from right, starting at 0)
unsigned int mask = 1 << n;
num ^= mask; // Toggle the nth bit
- Checking if a Bit is Set: You can use the bitwise AND operator (&) to check if a specific bit is set.
Syntax:
// Check if the nth bit (indexing from right, starting at 0) is set
unsigned int mask = 1 << n;
if (num & mask) {
// Bit is set
}
Get a comprehensive understanding of Recursion in Data Structure with our in-depth blog post!
Use Cases of Bitwise Operators
Here are the use cases of bitwise operators presented as bullet points:
- Cryptography and Hashing: Utilizes bitwise operations for encryption, decryption, and hashing algorithms
- Image and Multimedia Processing: Efficiently manipulate image pixels, color channels, and multimedia data
- Hardware Interaction: Interacts with hardware components by reading or modifying individual bits
- Data Storage Optimization: Used in algorithms like Huffman Coding and Run-Length Encoding
Career Transition
Conclusion
As the landscape of programming evolves, the core concepts of bitwise operators will remain relevant due to their efficiency, precision, and versatile applications. Future data scientists and algorithm developers can benefit from mastering these operations to craft efficient solutions across various problem domains.
Do you still have queries? You can post your doubts on our Community Page.
The post Bitwise Operators in C Programming appeared first on Intellipaat Blog.
Blog: Intellipaat - Blog
Leave a Comment
You must be logged in to post a comment.